Python Dictionary Append: Key/Value Pair
A dictionary is a collection of key-value pairs, where each key is unique and maps to a value. To append a new key-value pair to a dictionary in Python, you can use any of the following methods:
Using square bracket notation
The most common way to append a new key-value pair to a dictionary is by using square bracket notation.
In the above example, first create a dictionary called my_dict with two key-value pairs. Then append a new key-value pair to the dictionary using square bracket notation. The key is "gender" and the value is "Male". Finally, print the updated dictionary.
Using the update() method
Another way to append a new key-value pair to a dictionary is by using the update() method.
In the above example, first create a dictionary called my_dict with two key-value pairs. Then use the update() method to append a new key-value pair to the dictionary. The argument to the update() method is another dictionary with the new key-value pair. Finally, print the updated dictionary.
Using the dict() constructor
You can also use the dict() constructor to create a new dictionary with the existing key-value pairs and the new key-value pair.
In the above example, first create a dictionary called my_dict with two key-value pairs. Then use the dict() constructor to create a new dictionary called new_dict. Pass my_dict as the first argument to the constructor and the new key-value pair as keyword arguments. Finally, print the updated dictionary.
Using the setdefault() method
The setdefault() method is another way to append a new key-value pair to a dictionary.
In the above example, first create a dictionary called my_dict with two key-value pairs. Then use the setdefault() method to append a new key-value pair to the dictionary. The first argument to the setdefault() method is the key, and the second argument is the default value to use if the key doesn't exist in the dictionary. Finally, print the updated dictionary.
Adding to a Dictionary Using the Merge | Operator
In Python 3.9 and later versions, you can use the merge operator to add new key-value pairs to a dictionary.
In the above example, first create a dictionary called my_dict with two key-value pairs. Then, add a new key-value pair to the dictionary using the merge operator. The left-hand side of the operator is the dictionary to be updated, and the right-hand side is another dictionary with the new key-value pair. Finally, print the updated dictionary.
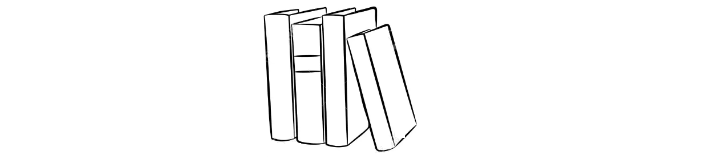
Note that this method is essentially the same as update() method mentioned above. Both methods use the update() method to add a new key-value pair to a dictionary. The only difference is that in this method, use the merge operator, which is a more concise and readable way to update a dictionary in Python 3.9 and later versions.
Append values to an existing key to a dictionary
You can append a new value to an existing key in a dictionary by using the square bracket notation to access the key and then using the append() method to add the new value to the corresponding list.
In the above example, first create a dictionary called my_dict with two keys, "name" and "age", and their corresponding values as lists. Then, append a new value to the "name" key and a new value to the "age" key using the append() method. Finally, print the updated dictionary.
Note that this method only works if the existing value of the key is a list. If the existing value is not a list, you will get a TypeError.
Adding to a Dictionary Without Overwriting Values
To add new key-value pairs to a dictionary without overwriting existing values, you can use the setdefault() method or the defaultdict class from the collections module.
Using setdefault()
The setdefault() method returns the value of a key in a dictionary. If the key does not exist in the dictionary, it adds the key with the specified value to the dictionary.
Note that if the key already exists in the dictionary, setdefault() does not overwrite the existing value.
Using defaultdict
The defaultdict class is a subclass of the dict class that overrides one method, __missing__(), to provide a default value for a nonexistent key.
In thje above example, first create a dictionary called my_dict with two key-value pairs. Then, create a defaultdict called my_default_dict with a default value of "Male" and initialize it with the contents of my_dict. Add a new key-value pair to my_default_dict, and the default value is not used because the key already exists in the dictionary. Finally, print both dictionaries to show that my_dict has not been modified, and my_default_dict has the new key-value pair.
Note that the default value is only used when a key does not exist in the dictionary.