os.path.join() method | Python
In Python, os.path.join is a method provided by the built-in os module that is used to join one or more path components together to form a complete path. The method takes one or more arguments, each of which represents a path component, and returns a string that represents the full path.
How to Use Python os.path.join
The main purpose of os.path.join is to create platform-independent path names that work on different operating systems such as Windows, Linux, and macOS. This is because different operating systems use different path separators (e.g., Windows uses backslashes \, while Unix-based systems use forward slashes /).
Syntax:In the above syntax, component1, component2, ..., and componentN are the individual path components to be joined. The method returns a string that represents the full path formed by joining these components together.
For example, consider the following code:
In the above code, the os.path.join method is used to join the path components "home", "user", and "data.txt" to form a complete path. The resulting path is printed to the console.
Handling file and directory Paths in Python
Following are a few examples of how to use the os.path.join() method to join various path components in Python:
Joining path components to create a directory path:
In the above example, join four path components to create a relative directory path. Note that, use forward slashes to separate the path components, which is the convention in Unix-based systems.
Joining path components using variables:
In the above example, use variables to represent the path components, which makes the code more modular and easier to read. Then use the os.path.join() method to join the path components into a complete file path.
Python File Path
In Python, a file path is a string that specifies the location of a file or directory in the file system. A file path consists of one or more path components separated by a path separator character, which is different depending on the operating system. The exact structure of a file path depends on whether it is an absolute path or a relative path.
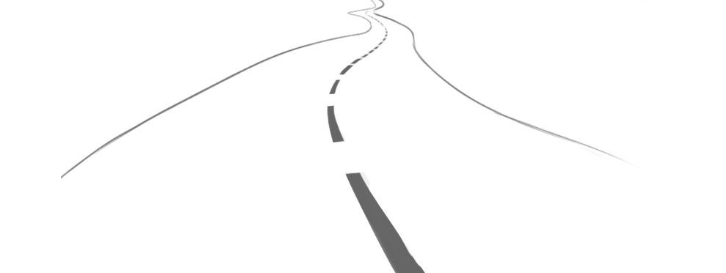
Absolute file path
An absolute file path specifies the exact location of a file or directory in the file system, starting from the root directory. The root directory is the top-level directory of the file system, and its name varies depending on the operating system. For example, on Windows, the root directory is typically C:\, while on Unix-based systems such as Linux and macOS, the root directory is /.
Following is an example of an absolute file path on a Unix-based system:
In the above code, the path components are / (root directory), home, user, data, and file.txt. The path specifies the exact location of the file file.txt in the data directory, which is located in the user directory, which is located in the home directory, which is located in the root directory.
Relative file path
A relative file path specifies the location of a file or directory relative to the current working directory. The current working directory is the directory in which the Python script is executed. A relative file path can use various path components such as .. to navigate up one level in the directory tree, or . to refer to the current directory.
Following is an example of a relative file path:
In the above code, the path components are .. (go up one level in the directory tree), data, and file.txt. The path specifies the location of the file file.txt in the data directory, which is located in the parent directory of the current working directory.
Python os module
The os module is a built-in module in Python that provides a way of using operating system dependent functionality, such as reading or writing to the file system, interacting with the network, and working with process management. It provides a consistent interface to interact with the underlying operating system, regardless of the specific operating system being used. Some commonly used functions in the os module include os.getcwd() to get the current working directory, os.listdir() to list the contents of a directory, os.path.join() to join one or more path components together, and os.system() to execute a shell command.
- Python print statement "Syntax Error: invalid syntax"
- Installing Python Modules with pip
- How to get current date and time in Python?
- No module named 'pip'
- How to get the length of a string in Python
- ModuleNotFoundError: No module named 'sklearn'
- ModuleNotFoundError: No module named 'cv2'
- Python was not found; run without arguments
- Attempted relative import with no known parent package
- TypeError: only integer scalar arrays can be converted to a scalar index
- A value is trying to be set on a copy of a slice from a DataFrame
- ValueError: setting an array element with a sequence
- Indentationerror: unindent does not match any outer indentation level
- Valueerror: if using all scalar values, you must pass an index
- ImportError: libGL.so.1: cannot open shared object file: No such file or directory
- Python Try Except | Exception Handling
- Custom Exceptions in Python with Examples
- Python String replace() Method
- sqrt Python | Find the Square Root in Python
- Read JSON file using Python
- Binary search in Python
- Defaultdict in Python
- Int Object is Not Iterable – Python Error
- TypeError: int object is not subscriptable
- Python multiline comment
- Typeerror: str object is not callable
- Python reverse List
- zip() in Python for Parallel Iteration
- strftime() in Python
- Typeerror: int object is not callable
- Python List pop() Method
- Fibonacci series in Python
- Python any() function
- Python any() Vs all()
- Python pass Statement
- Python Lowercase - String lower() Method
- Modulenotfounderror: no module named istutils.cmd
- Append to dictionary in Python : Key/Value Pair
- timeit | Measure execution time of small code
- Python Decimal to Binary
- GET and POST requests using Python
- Difference between List VS Set in Python
- How to Build Word Cloud in Python?
- Binary to Decimal in Python
- Modulenotfounderror: no module named 'apt_pkg'
- Convert List to Array Python