Read and Parse JSON file using Python
JSON (JavaScript Object Notation) is a lightweight data format that is easy for humans to read and write and easy for machines to parse and generate. In Python, the built-in json module provides methods for reading and parsing JSON data.
Following is an example of how to read and parse a JSON file in Python:
Sample JSON File
Suppose you have a data.json file containing the following JSON data:
Read and Parse JSON Files
You can read and parse this file using the following Python code:
In the above code, first import the json module. Then use the open() function to open the data.json file and the json.load() function to load the JSON data from the file into a Python Dictionary called data. Then access the data in the dictionary using keys, just like with any other Python dictionary.
Reading JSON from a File with Python json.loads()
If the JSON data is in a string instead of a file, you can use the json.loads() function instead of json.load().
In the above code, define the JSON data as a string instead of reading it from a file. Use the json.loads() function to parse the JSON data from the string into a Python Dictionary called data. You can then access the data in the dictionary using keys, just like before.
Use json.load() to parse nested objects or arrays
If the JSON data contains nested objects or arrays, you can access the data using indexing or looping, just like with any other Python data structure.
Suppose you have a data.json file containing the following JSON data:
You can read and parse this file using the following Python code:
In this code, first import the json module and use the open() function to open the data.json file. Then use the json.load() function to load the JSON data from the file into a Python dictionary called data. You can access the nested data in the dictionary using indexing or looping, just like with any other Python data structure. For example, you access the name of the first pet using data['pets'][0]['name'].
Python dictionary and JSON
Python dictionaries and JSON (JavaScript Object Notation) are closely related data structures.
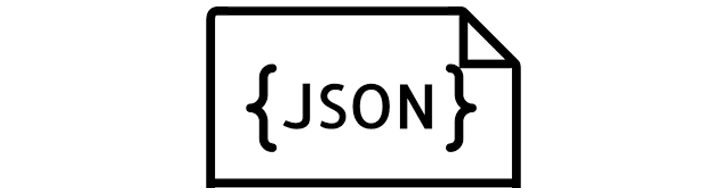
JSON is a lightweight data format that is easy for humans to read and write and easy for machines to parse and generate. It is based on a subset of the JavaScript programming language, but can be used in any programming language, including Python. Python dictionaries are a built-in data structure that allow you to store key-value pairs. Each key in a dictionary must be unique and immutable, while values can be of any data type, including other dictionaries, lists, and tuples.
Convert the Python Dictionary to JSON
JSON data is often used to represent complex data structures, such as nested dictionaries and lists. In fact, JSON data is almost identical to a Python dictionary or list.
In this code, define a Python Dictionary called data that contains some key-value pairs, including a list of dictionaries. Then use the json.dumps() function to convert the dictionary to a JSON-formatted string.
Print JSON data using Python
You can print the JSON data using print().
The output should look like this:
JSON to a Python Dictionary
You can also convert JSON data back to a Python dictionary using the json.loads() function.
In the above code, define a JSON-formatted string called json_str. Then use the json.loads() function to convert the string to a Python dictionary called data. You can then access the data in the dictionary using keys, just like before.
- Python print statement "Syntax Error: invalid syntax"
- Installing Python Modules with pip
- How to get current date and time in Python?
- No module named 'pip'
- How to get the length of a string in Python
- ModuleNotFoundError: No module named 'sklearn'
- ModuleNotFoundError: No module named 'cv2'
- Python was not found; run without arguments
- Attempted relative import with no known parent package
- TypeError: only integer scalar arrays can be converted to a scalar index
- A value is trying to be set on a copy of a slice from a DataFrame
- ValueError: setting an array element with a sequence
- Indentationerror: unindent does not match any outer indentation level
- Valueerror: if using all scalar values, you must pass an index
- ImportError: libGL.so.1: cannot open shared object file: No such file or directory
- Python Try Except | Exception Handling
- Custom Exceptions in Python with Examples
- Python String replace() Method
- sqrt Python | Find the Square Root in Python
- Binary search in Python
- Defaultdict in Python
- Int Object is Not Iterable – Python Error
- os.path.join in Python
- TypeError: int object is not subscriptable
- Python multiline comment
- Typeerror: str object is not callable
- Python reverse List
- zip() in Python for Parallel Iteration
- strftime() in Python
- Typeerror: int object is not callable
- Python List pop() Method
- Fibonacci series in Python
- Python any() function
- Python any() Vs all()
- Python pass Statement
- Python Lowercase - String lower() Method
- Modulenotfounderror: no module named istutils.cmd
- Append to dictionary in Python : Key/Value Pair
- timeit | Measure execution time of small code
- Python Decimal to Binary
- GET and POST requests using Python
- Difference between List VS Set in Python
- How to Build Word Cloud in Python?
- Binary to Decimal in Python
- Modulenotfounderror: no module named 'apt_pkg'
- Convert List to Array Python