Attempted relative import with no known parent package
The "ImportError: attempted relative import with no known parent package" error occurs when you try to import a module using a relative import, but Python cannot find the module's parent package.
Module's location
A relative import is a way to import a module that is located in the same package or module as the importing module. Instead of specifying the absolute path of the module to be imported, you use a relative path that starts with a dot (.) or two dots (..) to indicate the module's location relative to the importing module.
Suppose you have the following directory structure:
If you want to import submodule.py from mymodule.py, you can use a relative import like this:
The dot before subpackage indicates that it is located in the same package as mymodule.py.
However, if you try to run mymodule.py directly (i.e., not as a module imported by another module), you may get the "ImportError: attempted relative import with no known parent package" error. This is because Python cannot determine the package that contains the module being imported.
How to Fix: "ImportError: attempted relative import with no known parent package"
To fix this error, you need to make sure that the module being imported is part of a package, and that the package is on the Python path. You can do this by adding an empty __init__.py file to the package's directory, and by making sure that the directory containing the package is on the Python path.
For example, if you have the following directory structure:
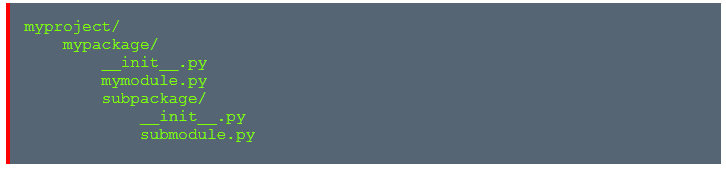
You can add the following lines to the beginning of mymodule.py to make the package and its subpackages available:
In the above example, first add the parent directory of mypackage to the Python path using os.path.abspath and os.path.join. Then, import submodule using a full import statement that specifies the package and submodule names.
Alternatively, you can run the module using the -m flag with the Python interpreter, like this:
This tells Python to run mymodule.py as a module, which will allow it to correctly resolve relative imports.
Absolute import Vs Relative import
In Python, packages are a way of organizing modules into a hierarchical structure. A package is simply a directory that contains one or more Python modules, along with an optional __init__.py file that is executed when the package is imported.
When importing modules from packages, there are two types of import paths: absolute and relative. The main difference between them is the way they specify the location of the module being imported.
Absolute import
An absolute import specifies the full path of the module being imported, starting from the root of the Python module search path. The search path is a list of directories where Python looks for modules when importing them. An absolute import always starts with the name of the package or module that contains the module being imported, and follows the package hierarchy using dot notation. For example:
In the above example, package1 is the top-level package, module1 is a module in package1, and function1 is a function defined in module1.
Relative import
A relative import, on the other hand, specifies the path of the module being imported relative to the location of the importing module. A relative import starts with one or two dots (. or ..) to indicate the level of the package hierarchy to traverse. For example:
In the above example, subpackage2 is a subpackage of the parent package of the importing module, and module2 is a module in subpackage2.
Advantage
The main advantage of using relative imports is that they make it easier to move packages and modules around within a project, without having to update all the import statements. They also make it easier to reuse code across multiple projects, because the import paths can be modified to match the package hierarchy of each project.
On the other hand, absolute imports provide a clear and unambiguous way of specifying the location of the module being imported, which can help avoid name clashes and make it easier to reason about the code. They are also the default behavior for imports in Python 3.x, and are recommended for most cases.
- Python print statement "Syntax Error: invalid syntax"
- Installing Python Modules with pip
- How to get current date and time in Python?
- No module named 'pip'
- How to get the length of a string in Python
- ModuleNotFoundError: No module named 'sklearn'
- ModuleNotFoundError: No module named 'cv2'
- Python was not found; run without arguments
- TypeError: only integer scalar arrays can be converted to a scalar index
- A value is trying to be set on a copy of a slice from a DataFrame
- ValueError: setting an array element with a sequence
- Indentationerror: unindent does not match any outer indentation level
- Valueerror: if using all scalar values, you must pass an index
- ImportError: libGL.so.1: cannot open shared object file: No such file or directory
- Python Try Except | Exception Handling
- Custom Exceptions in Python with Examples
- Python String replace() Method
- sqrt Python | Find the Square Root in Python
- Read JSON file using Python
- Binary search in Python
- Defaultdict in Python
- Int Object is Not Iterable – Python Error
- os.path.join in Python
- TypeError: int object is not subscriptable
- Python multiline comment
- Typeerror: str object is not callable
- Python reverse List
- zip() in Python for Parallel Iteration
- strftime() in Python
- Typeerror: int object is not callable
- Python List pop() Method
- Fibonacci series in Python
- Python any() function
- Python any() Vs all()
- Python pass Statement
- Python Lowercase - String lower() Method
- Modulenotfounderror: no module named istutils.cmd
- Append to dictionary in Python : Key/Value Pair
- timeit | Measure execution time of small code
- Python Decimal to Binary
- GET and POST requests using Python
- Difference between List VS Set in Python
- How to Build Word Cloud in Python?
- Binary to Decimal in Python
- Modulenotfounderror: no module named 'apt_pkg'
- Convert List to Array Python