How to convert a list to an array in Python
In Python, a list is a collection of elements that can be of any type, whereas an array is a collection of elements of the same type. Therefore, sometimes it may be necessary to convert a list to an array. You can use various methods to convert a list to an array in Python.
Using the array() function from the array module
The easiest way to convert a list to an array is to use the array() function from the array module in Python. The array() function creates an array of specified type and size from a Python list.
Following is the code to convert a list to an array using the array() function:
In the above code, 'i' is the type code for integer values. The array() function takes two arguments - the first argument is the type code for the array, and the second argument is the Python list that needs to be converted.
Using the numpy module
Another way to convert a list to an array is to use the numpy module in Python. The numpy module provides support for multidimensional arrays and matrices.
Following is the code to convert a list to an array using the numpy module:
In the above code, first import the numpy module and create a list of integers. Then use the array() function of numpy to convert the list to an array.
Using the fromlist() method of the array module
You can also use the fromlist() method of the array module to convert a list to an array. The fromlist() method creates an array from a Python list.
Following is the code to convert a list to an array using the fromlist() method:
In the above code, first import the array module and create a list of integers. Then create an empty array using the array() function and use the fromlist() method to populate the array with the elements of the list.
Using the append() method of the array module
You can also use the append() method of the array module to convert a list to an array. The append() method appends elements to the end of the array.
Following is the code to convert a list to an array using the append() method:
In the above code, first import the array module and create a list of integers. Then create an empty array using the array() function and use a for loop to append each element of the list to the end of the array.
Convert array of floating-point values
You can also use other data type codes to convert a list of elements to an array of different types. For example, if you have a list of floating-point values, you can use the data type code 'f' to convert it to an array of floating-point values:
In the above code, create a list of floating-point values and use the data type code 'f' to convert it to an array of floating-point values.
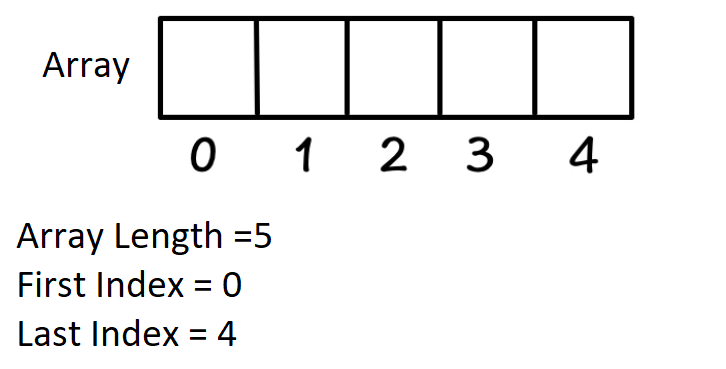
Here are some of the commonly used data type codes for the array() function:
- 'b': signed integer of size 1 byte (values between -128 and 127)
- 'B': unsigned integer of size 1 byte (values between 0 and 255)
- 'i': signed integer of size 2 bytes (values between -32,768 and 32,767)
- 'I': unsigned integer of size 2 bytes (values between 0 and 65,535)
- 'f': floating-point value of size 4 bytes
- 'd': floating-point value of size 8 bytes
- Python print statement "Syntax Error: invalid syntax"
- Installing Python Modules with pip
- How to get current date and time in Python?
- No module named 'pip'
- How to get the length of a string in Python
- ModuleNotFoundError: No module named 'sklearn'
- ModuleNotFoundError: No module named 'cv2'
- Python was not found; run without arguments
- Attempted relative import with no known parent package
- TypeError: only integer scalar arrays can be converted to a scalar index
- A value is trying to be set on a copy of a slice from a DataFrame
- ValueError: setting an array element with a sequence
- Indentationerror: unindent does not match any outer indentation level
- Valueerror: if using all scalar values, you must pass an index
- ImportError: libGL.so.1: cannot open shared object file: No such file or directory
- Python Try Except | Exception Handling
- Custom Exceptions in Python with Examples
- Python String replace() Method
- sqrt Python | Find the Square Root in Python
- Read JSON file using Python
- Binary search in Python
- Defaultdict in Python
- Int Object is Not Iterable – Python Error
- os.path.join in Python
- TypeError: int object is not subscriptable
- Python multiline comment
- Typeerror: str object is not callable
- Python reverse List
- zip() in Python for Parallel Iteration
- strftime() in Python
- Typeerror: int object is not callable
- Python List pop() Method
- Fibonacci series in Python
- Python any() function
- Python any() Vs all()
- Python pass Statement
- Python Lowercase - String lower() Method
- Modulenotfounderror: no module named istutils.cmd
- Append to dictionary in Python : Key/Value Pair
- timeit | Measure execution time of small code
- Python Decimal to Binary
- GET and POST requests using Python
- Difference between List VS Set in Python
- How to Build Word Cloud in Python?
- Binary to Decimal in Python
- Modulenotfounderror: no module named 'apt_pkg'