Multiline comments in Python
Python comments are annotations or notes that are used to explain the purpose or functionality of a piece of code.
In Python, there are two ways to write multi-line comments:
- Starting each line with the # character, which indicates that the entire line is a comment.
- Enclosing the comment in a set of triple quotes """ """ or ''' '''.
Consecutive single-line comment
You can create multi-line comments by starting each line with the # character. This is a simple and easy way to add comments to your code, and is useful when you want to quickly add a comment to multiple lines of code.
Following is an example of using the # character to create a multi-line comment:
In the above example, each line starts with the # character, which tells Python to treat the entire line as a comment. This is useful for adding comments to multiple lines of code, without having to enclose the entire block in triple quotes.
Using a Multi-line string as a comment
Follwoing is an example of using triple quotes to write a multi-line comment:
In the above code, the triple quotes """ """ or ''' ''' enclose a multi-line comment. Anything inside the triple quotes will be treated as a comment, even if it spans multiple lines.
Docstrings (Documentation Strings)
Triple quotes are also commonly used to write documentation strings (docstrings) for functions, classes, and modules. Following is an example:
In the above example, the triple quotes enclose a docstring that describes what the add_numbers() function does, what arguments it takes, and what it returns. Docstrings are used to document the purpose and usage of a function or module, and can be accessed using the __doc__ attribute.
Multi-line comments and docstrings are important tools for writing clear and maintainable code. They provide explanations and context that can help other programmers understand your code and make it easier to maintain over time.
Single line comment in Python
In Python, single-line comments are denoted by the hash character (#). Any text that follows the hash character on a line is ignored by the Python interpreter.
In the above example, the first line is a single-line comment that does not affect the output of the program. The second line is a Python statement that prints the text "Hello, World!" to the console, and the comment that follows the statement is also ignored by the interpreter.
Python Comments
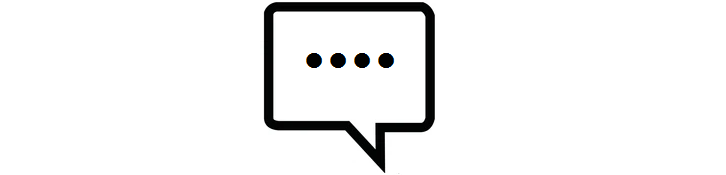
In Python, single-line comments are denoted by the hash symbol (#), while multi-line comments can be enclosed in triple quotes (''' ''') or within parentheses with the hash symbol (#) at the beginning of each line. Comments in Python are ignored by the interpreter and do not affect the execution of the program.
- Python print statement "Syntax Error: invalid syntax"
- Installing Python Modules with pip
- How to get current date and time in Python?
- No module named 'pip'
- How to get the length of a string in Python
- ModuleNotFoundError: No module named 'sklearn'
- ModuleNotFoundError: No module named 'cv2'
- Python was not found; run without arguments
- Attempted relative import with no known parent package
- TypeError: only integer scalar arrays can be converted to a scalar index
- A value is trying to be set on a copy of a slice from a DataFrame
- ValueError: setting an array element with a sequence
- Indentationerror: unindent does not match any outer indentation level
- Valueerror: if using all scalar values, you must pass an index
- ImportError: libGL.so.1: cannot open shared object file: No such file or directory
- Python Try Except | Exception Handling
- Custom Exceptions in Python with Examples
- Python String replace() Method
- sqrt Python | Find the Square Root in Python
- Read JSON file using Python
- Binary search in Python
- Defaultdict in Python
- Int Object is Not Iterable – Python Error
- os.path.join in Python
- TypeError: int object is not subscriptable
- Typeerror: str object is not callable
- Python reverse List
- zip() in Python for Parallel Iteration
- strftime() in Python
- Typeerror: int object is not callable
- Python List pop() Method
- Fibonacci series in Python
- Python any() function
- Python any() Vs all()
- Python pass Statement
- Python Lowercase - String lower() Method
- Modulenotfounderror: no module named istutils.cmd
- Append to dictionary in Python : Key/Value Pair
- timeit | Measure execution time of small code
- Python Decimal to Binary
- GET and POST requests using Python
- Difference between List VS Set in Python
- How to Build Word Cloud in Python?
- Binary to Decimal in Python
- Modulenotfounderror: no module named 'apt_pkg'
- Convert List to Array Python