Setting an array element with a sequence
ValueError: setting an array element with a sequence is an error message that can occur when you try to assign a sequence (such as a list or an array) to an element in a numpy array, but the sequence has a different shape than the element. In other words, the sequence you're trying to assign doesn't match the size and shape of the element in the array.
Mixing Arrays of Different Dimensions
Following is an example to illustrate this error:
In the above example, trying to assign the one-dimensional array b to the first row of a. However, b has a different shape than the first row of a, which is a one-dimensional array of length 2. This will result in a ValueError with the message "setting an array element with a sequence".
To fix this error, you need to make sure that the shape of the sequence you're trying to assign matches the shape of the element in the array. In the example above, you could fix the error by reshaping b to have the same shape as the first row of a:
In the above example, you have reshaped b to be a one-dimensional array of length 2, which matches the shape of the first row of a. Now the assignment works without error.
Use an object data type
In order to prevent the "ValueError: setting an array element with a sequence " error when trying to replace a single element of a NumPy array with an array of a different size, you can use an object data type. This allows the elements of the array to be any Python object, including arrays of different sizes.
Following is an example of creating an array with an object data type and replacing a single element with an array of a different size:
In the above example, create an array with an object data type using the np.empty() function. This creates an array with uninitialized elements that can be any Python object. Then print the arr array to verify its contents.
Then create a 1x4 array of ones using the np.ones() function and print it to verify its contents.
Then replace the first element of arr with ones_arr_4 by assigning ones_arr_4 to arr[0,0]. This operation works because arr[0,0] can be any Python object, including an array of any size.
Finally, print the arr array again to verify that the first element now contains the ones_arr_4 array.Note that using an object data type can have performance implications, as the elements of the array are not guaranteed to be stored contiguously in memory. If performance is a concern, it may be better to reshape the replacement array to match the size of the element being replaced or use a different data structure.
Replace a Single Array Element with an Array
When you try to replace a single element of the arr array with an array of a different size, you would get the "ValueError: setting an array element with a sequence" error.
Above code will raise a "ValueError: setting an array element with a sequence" error because ones_arr_4 has four elements, but we are trying to replace a single element of arr.
In Python, it is possible to replace a single element of an array with another array, but this operation is only valid if the replacement array has the same number of elements as the original array. Otherwise, a "ValueError: setting an array element with a sequence" error will be raised.
Following is an example of replacing a single element of a NumPy array with another array of the same size:
In the above example, first create a 3x3 array of zeros using the np.zeros() function. Then create a 1x3 array of ones using the np.ones() function and print both arrays to verify their contents.
Then replace the second row of the arr array with the ones_arr array by assigning ones_arr to the second element of arr. This operation works because ones_arr has the same number of elements as the row you are replacing.
Other methods:
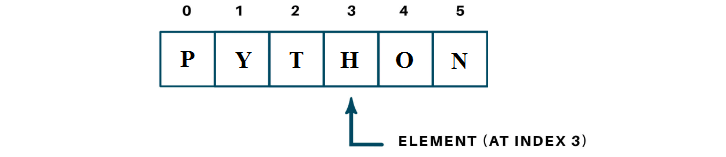
There are a few different methods you can use to solve the ValueError: setting an array element with a sequence error in NumPy.
Reshape the sequence
Reshape the sequence to match the shape of the array element:
One of the easiest ways to fix the error is to reshape the sequence so that it matches the shape of the array element you are trying to assign it to.
In the above example, you have reshaped the b array to be a one-dimensional array of length 2, which matches the shape of the first row of a. Now the assignment works without error.
Use indexing
Use indexing to assign each element of the sequence to the corresponding element in the array:
Another way to fix the error is to use indexing to assign each element of the sequence to the corresponding element in the array.
In the above example, you have used indexing to assign the elements of b to the first row of a. The : means that we are assigning the entire row, and the 0 means that you are assigning to the first row. Now the assignment works without error.
In general, the best method to use will depend on the specifics of your code and the shape of the arrays you are working with.
- Python print statement "Syntax Error: invalid syntax"
- Installing Python Modules with pip
- How to get current date and time in Python?
- No module named 'pip'
- How to get the length of a string in Python
- ModuleNotFoundError: No module named 'sklearn'
- ModuleNotFoundError: No module named 'cv2'
- Python was not found; run without arguments
- Attempted relative import with no known parent package
- TypeError: only integer scalar arrays can be converted to a scalar index
- A value is trying to be set on a copy of a slice from a DataFrame
- Indentationerror: unindent does not match any outer indentation level
- Valueerror: if using all scalar values, you must pass an index
- ImportError: libGL.so.1: cannot open shared object file: No such file or directory
- Python Try Except | Exception Handling
- Custom Exceptions in Python with Examples
- Python String replace() Method
- sqrt Python | Find the Square Root in Python
- Read JSON file using Python
- Binary search in Python
- Defaultdict in Python
- Int Object is Not Iterable – Python Error
- os.path.join in Python
- TypeError: int object is not subscriptable
- Python multiline comment
- Typeerror: str object is not callable
- Python reverse List
- zip() in Python for Parallel Iteration
- strftime() in Python
- Typeerror: int object is not callable
- Python List pop() Method
- Fibonacci series in Python
- Python any() function
- Python any() Vs all()
- Python pass Statement
- Python Lowercase - String lower() Method
- Modulenotfounderror: no module named istutils.cmd
- Append to dictionary in Python : Key/Value Pair
- timeit | Measure execution time of small code
- Python Decimal to Binary
- GET and POST requests using Python
- Difference between List VS Set in Python
- How to Build Word Cloud in Python?
- Binary to Decimal in Python
- Modulenotfounderror: no module named 'apt_pkg'
- Convert List to Array Python