sqrt Python | Find the Square Root in Python
The math.sqrt() method in Python is a built-in function of the math module, which is used to find the square root of a given number. This method takes a single argument, which is the number whose square root is to be calculated. It returns the square root of the input number as a floating-point value.
Syntax:
- x is the number whose square root is to be calculated
- result is the variable that stores the computed square root
Find the square root of a real number:
Find the square root of a negative real number:
Here, you get a ValueError because the square root of a negative number is not a real number.
Find Square root in Python using the cmath module
The cmath module in Python provides functions for complex number math, including computing the square root of complex numbers. The cmath.sqrt() function can be used to compute the square root of a complex number.
Following is an example of how to use cmath.sqrt() to calculate the square root of a complex number:
In the above example, first import the cmath module. Then define a complex number z with the value 2 + 3j. Use the cmath.sqrt() function to calculate the square root of z and store the result in the variable result. Finally, print the result, which is (1.6741492280355401+0.8959774761298381j).
Note that the result of cmath.sqrt() is a complex number, even if the input is a real number. If you want to convert the result back to a real number, you can use the abs() function to compute the magnitude of the complex number.
In the above example, compute the square root of the real number 25 using cmath.sqrt(), which returns a complex number. Then use the abs() function to compute the magnitude of the complex number, which is the same as the absolute value of the real part. The result is 5.0, which is the square root of 25.
Square root of a negative real number
Finding the square root of a negative real number using complex numbers:
Here, use the c math.sqrt() method to find the square root of a negative number using complex numbers. The result is a complex number with an imaginary part of 5j.
Find Square root in Python using pow() function
In Python, you can use the pow() function to calculate the square root of a number by raising it to the power of 0.5.
In this example, calculate the square root of the number 25 by raising it to the power of 0.5 using the pow() function. The result is 5.0, which is the square root of 25.
Note that this method only works for real numbers. If you want to calculate the square root of a negative number or a complex number, you should use the cmath.sqrt() function from the cmath module instead.
Find the square root in using the exponent operator
In Python, you can use the exponent operator (**) to calculate the square root of a number by raising it to the power of 0.5, just like with the pow() function.
In this example, calculate the square root of the number 25 by raising it to the power of 0.5 using the exponent operator (**). The result is 5.0, which is the square root of 25.
The Python Square Root Function
The math.sqrt() method can be used in a variety of applications, including:
Find the length of the hypotenuse of a right-angled triangle:
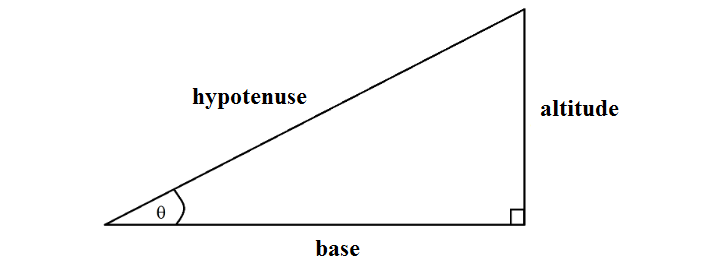
In a right-angled triangle, the length of the hypotenuse (the longest side) can be calculated using the Pythagorean theorem. The theorem states that the square of the hypotenuse is equal to the sum of the squares of the other two sides. Using the math.sqrt() method, we can calculate the length of the hypotenuse as follows:
Find the distance between two points:
The distance between two points in a two-dimensional plane can be calculated using the distance formula, which involves the square root of the sum of the squares of the differences between the coordinates. Using the math.sqrt() method, we can calculate the distance between two points as follows:
Calculating the standard deviation of a dataset:
The standard deviation is a measure of the amount of variation or dispersion in a dataset. It is calculated as the square root of the variance, which is the average of the squared differences from the mean. Using the math.sqrt() method, you can can calculate the standard deviation of a dataset as follows:
Calculating the magnitude of a vector:
The magnitude of a vector is the square root of the sum of the squares of its components. Using the math.sqrt() method, we can calculate the magnitude of a vector as follows:
- Python print statement "Syntax Error: invalid syntax"
- Installing Python Modules with pip
- How to get current date and time in Python?
- No module named 'pip'
- How to get the length of a string in Python
- ModuleNotFoundError: No module named 'sklearn'
- ModuleNotFoundError: No module named 'cv2'
- Python was not found; run without arguments
- Attempted relative import with no known parent package
- TypeError: only integer scalar arrays can be converted to a scalar index
- A value is trying to be set on a copy of a slice from a DataFrame
- ValueError: setting an array element with a sequence
- Indentationerror: unindent does not match any outer indentation level
- Valueerror: if using all scalar values, you must pass an index
- ImportError: libGL.so.1: cannot open shared object file: No such file or directory
- Python Try Except | Exception Handling
- Custom Exceptions in Python with Examples
- Python String replace() Method
- Read JSON file using Python
- Binary search in Python
- Defaultdict in Python
- Int Object is Not Iterable – Python Error
- os.path.join in Python
- TypeError: int object is not subscriptable
- Python multiline comment
- Typeerror: str object is not callable
- Python reverse List
- zip() in Python for Parallel Iteration
- strftime() in Python
- Typeerror: int object is not callable
- Python List pop() Method
- Fibonacci series in Python
- Python any() function
- Python any() Vs all()
- Python pass Statement
- Python Lowercase - String lower() Method
- Modulenotfounderror: no module named istutils.cmd
- Append to dictionary in Python : Key/Value Pair
- timeit | Measure execution time of small code
- Python Decimal to Binary
- GET and POST requests using Python
- Difference between List VS Set in Python
- How to Build Word Cloud in Python?
- Binary to Decimal in Python
- Modulenotfounderror: no module named 'apt_pkg'
- Convert List to Array Python