Typeerror: str object is not callable
The TypeError: 'str' object is not callable error occurs when you try to call a string object as if it were a function. This can happen due to several reasons, such as overwriting a built-in function with a string, having a function and a variable with the same name, or calling a function that returns a string twice. To fix this error, you need to identify the cause of the error and correct the issue.
Let's take a look at some examples of common causes for the TypeError: 'str' object is not callable error:
Attempting to call a string with parentheses
This error can occur if you try to call a string with parentheses as if it were a function.
In the above case, greeting is a string, not a function, so attempting to call it like a function will result in a TypeError.
The correct way to print the string would be:
Overwriting a built-in function with a string
Another possible cause of this error is overwriting a built-in function with a string.
In the above case, str is a built-in function in Python that converts an object to a string. However, you have overwritten it with a string object, so attempting to call it like a function will result in a TypeError.
To fix this, you can simply choose a different name for our string variable:
Using parentheses after a variable name
Also, this error can also occur if you accidentally put parentheses after a variable name, making Python think you are trying to call a function.
In the above case, name is a string, not a function, so attempting to call it like a function will result in a TypeError.
To fix this, you can simply remove the parentheses:
Having a function and a variable with the same name
If you have a function and a variable with the same name, it can cause the TypeError: 'str' object is not callable error. This is because when you call the name as a function, Python will try to use the variable instead of the function, resulting in a TypeError.
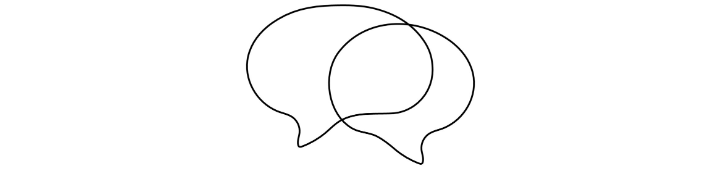
For example, suppose you have a function called foo() and a variable with the same name:
In the above case, when you try to call foo() as a function, Python will try to use the variable instead, resulting in a TypeError.
To fix this, you can rename the variable to something different:
In the above updated code, renamed the variable to foo_var, which prevents the name conflict and allows you to call the function without errors.
Alternatively, you can also rename the function to something different if the variable is used more frequently or is critical to your program. In general, it's best practice to avoid using the same name for functions and variables to prevent these types of errors.
- Python print statement "Syntax Error: invalid syntax"
- Installing Python Modules with pip
- How to get current date and time in Python?
- No module named 'pip'
- How to get the length of a string in Python
- ModuleNotFoundError: No module named 'sklearn'
- ModuleNotFoundError: No module named 'cv2'
- Python was not found; run without arguments
- Attempted relative import with no known parent package
- TypeError: only integer scalar arrays can be converted to a scalar index
- A value is trying to be set on a copy of a slice from a DataFrame
- ValueError: setting an array element with a sequence
- Indentationerror: unindent does not match any outer indentation level
- Valueerror: if using all scalar values, you must pass an index
- ImportError: libGL.so.1: cannot open shared object file: No such file or directory
- Python Try Except | Exception Handling
- Custom Exceptions in Python with Examples
- Python String replace() Method
- sqrt Python | Find the Square Root in Python
- Read JSON file using Python
- Binary search in Python
- Defaultdict in Python
- Int Object is Not Iterable – Python Error
- os.path.join in Python
- TypeError: int object is not subscriptable
- Python multiline comment
- Python reverse List
- zip() in Python for Parallel Iteration
- strftime() in Python
- Typeerror: int object is not callable
- Python List pop() Method
- Fibonacci series in Python
- Python any() function
- Python any() Vs all()
- Python pass Statement
- Python Lowercase - String lower() Method
- Modulenotfounderror: no module named istutils.cmd
- Append to dictionary in Python : Key/Value Pair
- timeit | Measure execution time of small code
- Python Decimal to Binary
- GET and POST requests using Python
- Difference between List VS Set in Python
- How to Build Word Cloud in Python?
- Binary to Decimal in Python
- Modulenotfounderror: no module named 'apt_pkg'
- Convert List to Array Python