TypeError: int object is not subscriptable
The error message "int object is not subscriptable" typically occurs in Python when you try to use the square bracket notation to access a particular element of an integer (or any other non-indexable data type).
An Example Scenario
Following is an example to illustrate this error:
When you run this code, you'll get the following error message:
This error message means that you can't use the square bracket notation to access a particular element of the integer 'x', because integers are not indexable or subscriptable.
How to fix: 'int' object is not subscriptable
Following are some ways to fix this error:
Convert the integer to a string:
If you want to access a specific digit of an integer, you can convert the integer to a string using the str() function and then use square bracket notation to access the desired digit.
Check that you are using the correct data type:
Make sure that the variable you are trying to access with square brackets is an indexable data type such as a list, tuple, or string.
Check that you are not trying to access an index that doesn't exist:
If you are trying to access an index of a list or tuple, make sure that the index you are trying to access actually exists in the list or tuple.
Use conditional statements:
If you want to access a specific element of a list or tuple based on a condition, use conditional statements instead of square bracket notation.
By following these steps, you can fix the "int object is not subscriptable" error in Python.
Subscriptable objects in Python
In Python, subscriptable objects are those that can be accessed using the square bracket notation. These include:
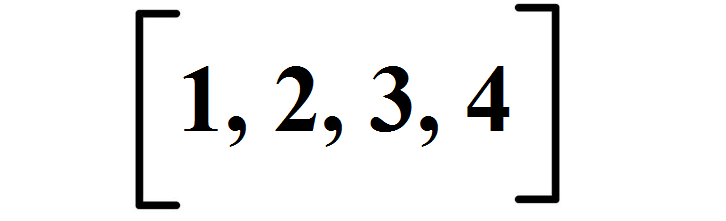
Lists:
A list is a collection of items, which can be of any data type, and are enclosed in square brackets []. Items in a list can be accessed using the square bracket notation and an index.
Tuples:
A tuple is similar to a list, but it is immutable (cannot be changed) and is enclosed in parentheses (). Items in a tuple can be accessed using the square bracket notation and an index.
Strings:
A string is a collection of characters, enclosed in either single or double quotes. Characters in a string can be accessed using the square bracket notation and an index.
Dictionaries:
A dictionary is a collection of key-value pairs, enclosed in curly braces {}. Values in a dictionary can be accessed using the square bracket notation and a key.
Sets:
A set is a collection of unique items, enclosed in curly braces {}. Items in a set cannot be accessed using an index, but some set operations like "in" or "not in" can be used to check for the presence of an item.
Subscriptable objects are an important concept in Python and are used frequently in programming to access and manipulate data.
- Python print statement "Syntax Error: invalid syntax"
- Installing Python Modules with pip
- How to get current date and time in Python?
- No module named 'pip'
- How to get the length of a string in Python
- ModuleNotFoundError: No module named 'sklearn'
- ModuleNotFoundError: No module named 'cv2'
- Python was not found; run without arguments
- Attempted relative import with no known parent package
- TypeError: only integer scalar arrays can be converted to a scalar index
- A value is trying to be set on a copy of a slice from a DataFrame
- ValueError: setting an array element with a sequence
- Indentationerror: unindent does not match any outer indentation level
- Valueerror: if using all scalar values, you must pass an index
- ImportError: libGL.so.1: cannot open shared object file: No such file or directory
- Python Try Except | Exception Handling
- Custom Exceptions in Python with Examples
- Python String replace() Method
- sqrt Python | Find the Square Root in Python
- Read JSON file using Python
- Binary search in Python
- Defaultdict in Python
- Int Object is Not Iterable – Python Error
- os.path.join in Python
- Python multiline comment
- Typeerror: str object is not callable
- Python reverse List
- zip() in Python for Parallel Iteration
- strftime() in Python
- Typeerror: int object is not callable
- Python List pop() Method
- Fibonacci series in Python
- Python any() function
- Python any() Vs all()
- Python pass Statement
- Python Lowercase - String lower() Method
- Modulenotfounderror: no module named istutils.cmd
- Append to dictionary in Python : Key/Value Pair
- timeit | Measure execution time of small code
- Python Decimal to Binary
- GET and POST requests using Python
- Difference between List VS Set in Python
- How to Build Word Cloud in Python?
- Binary to Decimal in Python
- Modulenotfounderror: no module named 'apt_pkg'
- Convert List to Array Python