Python Timeit() with Examples
Python's timeit module provides a way to measure the execution time of small code snippets . It can be used to determine the execution time of a single line of code or an entire function.
Syntax:
- stmt: This is the statement to be timed. It can be a single line of code or a multi-line code block.
- setup: This is a setup statement that is executed only once before the timed statement. It can be used to import modules or define variables that are used in the timed statement.
- timer: This is an optional timer function that can be used to measure the execution time. The default timer function is time.perf_counter.
- number: This is the number of times the statement is executed. The default value is number=1.
Following are some examples of how to use timeit:
Timing a single line of code
Above code snippet times the execution of a single line of code that sums the range of numbers from 0 to 99. The timeit.timeit function returns the execution time in seconds.
Timing a function call
Above code snippet times the execution of a function call that sums the range of numbers from 0 to 99. The setup parameter is used to import the function into the timer namespace so that it can be called from the timed statement.
Timing a multi-line code block using triple quotes
Above code snippet times the execution of a multi-line code block that sums the range of numbers from 0 to 99. The code block is defined as a string and passed to the timeit.timeit function as the timed statement.
Timing Multiple lines block using semicolon
You can use a semicolon to separate multiple statements on the same line. This can be useful for timing multiple lines of code with the timeit module. Here is an example:
Above code snippet times the execution of multiple statements separated by semicolons. The timeit.timeit function returns the execution time in seconds.
Note that using semicolons to separate statements on the same line can make the code harder to read and maintain. It's generally better to use separate lines for each statement, especially for longer or more complex code blocks.
Using a custom timer function
Above code snippet times the execution of a single line of code that sums the range of numbers from 0 to 99. The timer parameter is used to specify a custom timer function that measures CPU time instead of wall-clock time.
Other commonly used functions in timeit
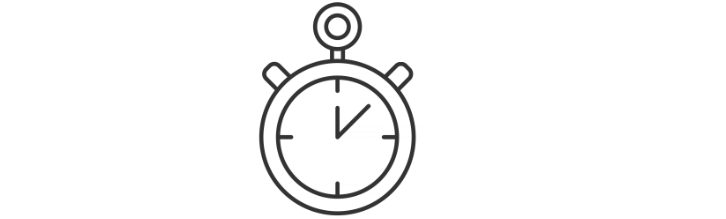
The timeit module in Python provides a way to time the execution of small bits of Python code. Here are some commonly used functions in the timeit module along with examples:
timeit.repeat(stmt, setup, timer, repeat, number)
This function is similar to timeit.timeit(), but it executes the statement multiple times and returns a list of timings for each execution. The repeat parameter specifies the number of times to execute the statement.
Repeating a simple statement:timeit.default_timer()
This function returns a timer function that is used by default in timeit.timeit() and timeit.repeat().
timeit.Timer(stmt, setup)
This class provides an alternative way to time a statement. It takes the statement and setup as parameters and provides methods to time the execution.
timeit.Timer(stmt, setup)
timeit.Timer(stmt, setup) is a class in the timeit module that provides an alternative way to time the execution of a statement. It takes two parameters:
- stmt (required): The statement to be timed as a string.
- setup (optional): The setup code to be executed before the statement as a string. If not provided, an empty string is used.
The Timer object has the following methods to time the execution of the statement:
- timeit(number=1000000): This method executes the statement number times and returns the total time taken in seconds.
- repeat(repeat=5, number=1000000): This method repeats the timeit method repeat times and returns a list of the timings.
The number parameter specifies the number of times to execute the statement for each timing.
In the above example, the Timer object is created with a simple setup and statement. The timeit method is called on the timer object to time the execution of the statement. The repeat method is called on the timer object to repeat the timing 3 times and return the timings.
timeit.time_per_call(stmt, setup=None, timer=This function is used to time a single call to the given statement stmt by executing it number times and returning the time taken per call.
timeit.Timer(stmt='pass', setup='pass', timer=, globals=None)
This class provides an alternative way to time a statement. It takes the statement and setup as parameters and provides methods to time the execution.
timeit.default_repeat()
This function returns the default value for the repeat parameter in timeit.repeat(). It is currently set to 5.
timeit.default_number()
This function returns the default value for the number parameter in timeit.timeit() and timeit.repeat(). It is currently set to 1000000.
timeit.disable_gc()
This function disables the garbage collector during timing. It can be used to get more accurate timings for code that creates a lot of garbage.
timeit.enable_gc()
This function enables the garbage collector during timing. It is enabled by default.
timeit.get_ident()
This function returns a unique integer identifier for the current thread.
timeit.Timer.autorange(callback=None)
This method is used to automatically determine the number of iterations to use for timing. It takes an optional callback function that can be used to control the timing process.
These are some of the commonly used functions in the timeit module in Python.
- Python print statement "Syntax Error: invalid syntax"
- Installing Python Modules with pip
- How to get current date and time in Python?
- No module named 'pip'
- How to get the length of a string in Python
- ModuleNotFoundError: No module named 'sklearn'
- ModuleNotFoundError: No module named 'cv2'
- Python was not found; run without arguments
- Attempted relative import with no known parent package
- TypeError: only integer scalar arrays can be converted to a scalar index
- A value is trying to be set on a copy of a slice from a DataFrame
- ValueError: setting an array element with a sequence
- Indentationerror: unindent does not match any outer indentation level
- Valueerror: if using all scalar values, you must pass an index
- ImportError: libGL.so.1: cannot open shared object file: No such file or directory
- Python Try Except | Exception Handling
- Custom Exceptions in Python with Examples
- Python String replace() Method
- sqrt Python | Find the Square Root in Python
- Read JSON file using Python
- Binary search in Python
- Defaultdict in Python
- Int Object is Not Iterable – Python Error
- os.path.join in Python
- TypeError: int object is not subscriptable
- Python multiline comment
- Typeerror: str object is not callable
- Python reverse List
- zip() in Python for Parallel Iteration
- strftime() in Python
- Typeerror: int object is not callable
- Python List pop() Method
- Fibonacci series in Python
- Python any() function
- Python any() Vs all()
- Python pass Statement
- Python Lowercase - String lower() Method
- Modulenotfounderror: no module named istutils.cmd
- Append to dictionary in Python : Key/Value Pair
- Python Decimal to Binary
- GET and POST requests using Python
- Difference between List VS Set in Python
- How to Build Word Cloud in Python?
- Binary to Decimal in Python
- Modulenotfounderror: no module named 'apt_pkg'
- Convert List to Array Python