Python Try Except | Exception Handling
In Python, an exception is an event that occurs during the execution of a program and disrupts the normal flow of the program's instructions. When an exception occurs, Python raises an object that represents the exception and halts the execution of the program unless the exception is handled by an appropriate exception handler.
Exception Handling | Python
There are many types of exceptions in Python, including built-in exceptions like TypeError, ValueError, ZeroDivisionError, and NameError, among others. Each of these exceptions represents a specific type of error that can occur during program execution.
For example, a TypeError exception is raised when an operation or function is applied to an object of inappropriate type, whereas a ValueError exception is raised when a built-in operation or function receives an argument that has the right type but an inappropriate value.
In Python, you can use a try-except block to handle exceptions. The try block contains the code that may raise an exception, and the except block contains the code that handles the exception. By handling exceptions , you can avoid program crashes and ensure that your program continues to execute despite any errors that occur.
Try and Except in Python
Python has try-except statements which are used for error handling. The syntax for a try-except block is:
Here, try is the keyword that starts the block of code that might raise an exception, and except is the keyword that starts the block of code that will handle the exception. The ExceptionType is the type of exception that you want to catch. If an exception of that type is raised in the try block, then the code in the except block will be executed.
Multiple except blocks in Python
You can also have multiple except blocks to catch different types of exceptions, or an else block to run code that should be executed if no exception was raised in the try block, and a finally block to run code that should be executed regardless of whether an exception was raised or not.
The try block contains the code that may raise an exception. The except blocks contain the code that handles the exceptions of different types. If an exception of a specific type occurs in the try block, the corresponding except block will be executed to handle that exception. The else block contains code that is executed if no exception was raised in the try block. The finally block contains code that is executed regardless of whether an exception was raised or not.
Python Exception Handling | Example
When opening a file in Python, there are several types of exceptions that can occur, such as FileNotFoundError if the file does not exist, PermissionError if the file cannot be opened due to permission issues, and IOError if there is a problem reading or writing the file. To handle these exceptions, you can use a try-except block with multiple except clauses.
In the above example, use the with statement to open the file in read mode, and perform some operation with the file inside the with block. If any of the specified exceptions occur during this operation, the corresponding except block is executed.
Catch Multiple Exceptions in Python
The first except block handles the FileNotFoundError exception, which is raised if the specified file does not exist. The second except block handles the PermissionError exception, which is raised if the file cannot be opened due to permission issues. The third except block handles the IOError exception, which is a general exception that can be raised if there is a problem reading or writing the file. In this block, print a more detailed error message using the errno and strerror attributes of the IOError object.
Try, Except, else and Finally in Python
Additionally, have an else clause, which is executed if no exceptions are raised during the file operations. In this case, you can print a message indicating that the file was opened successfully. Also have a finally clause, which is always executed regardless of whether an exception occurred or not. In this block, you can perform any necessary cleanup operations to release resources that were used during the file operations. In this example, simply print a message indicating that cleaning up resources.
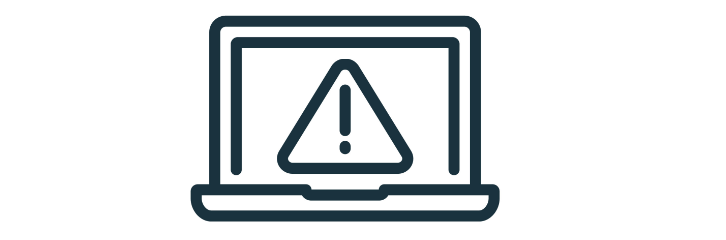
User-Defined Exceptions in Python
In addition to built-in exceptions, Python also allows you to define your own exceptions by creating a new exception class. User-defined exceptions (custom exceptions) can be useful when you want to create an exception type that is specific to your program or module.
To create a new exception class, you can define a new class that inherits from the built-in Exception class or one of its subclasses. For example, you could define a new exception class called MyException as follows:
In the above example, MyException is a new class that inherits from the built-in Exception class. The pass statement is used as a placeholder for the class definition, since you don't need to define any additional methods or attributes for this exception class.
Once you have defined a new exception class, you can raise instances of that class using the raise keyword, just like you would with a built-in exception. For example, you could raise an instance of MyException like this:
In the above example, you raise an instance of MyException with the message "Something went wrong". This will cause the program to halt and search for an appropriate exception handler.
You can also define custom methods and attributes for your exception class, just like you would with any other class. For example, you could define a custom method for MyException that returns a formatted error message:
In the above example, define a custom __init__ method that takes a message as an argument and saves it as an attribute of the instance. Also define a custom __str__ method that formats the error message as a string. This allows us to raise instances of MyException with a custom error message, and have that message formatted in a specific way.
Raising an Exception in Python
In Python, you can raise an exception manually using the raise keyword. Raising an exception manually can be useful in situations where you want to indicate that an error has occurred and stop the execution of the program.
To raise an exception manually, you can use the following syntax:
Here, ExceptionType is the type of exception you want to raise, and "Error message" is the message you want to associate with the exception.
For example, suppose you have a function that takes an argument and raises an exception if the argument is not a positive integer. You could define the function as follows:
In the above example, if the argument x is not a positive integer, the function raises a ValueError exception with the message "Argument must be a positive integer". If the argument x is a positive integer, the function returns the value of x.
When you raise an exception manually, Python searches for an appropriate exception handler to handle the exception. If an appropriate handler is not found, the program terminates with an error message. If an appropriate handler is found, the program continues to execute after the exception is handled.
Conclusion:
Python provides a wide range of built-in exception types, and you can also define your own custom exceptions to handle specific types of errors in your program. By using try-except blocks, you can ensure that your program handles exceptions elegantly and continues to execute even if an error occurs.
- Python print statement "Syntax Error: invalid syntax"
- Installing Python Modules with pip
- How to get current date and time in Python?
- No module named 'pip'
- How to get the length of a string in Python
- ModuleNotFoundError: No module named 'sklearn'
- ModuleNotFoundError: No module named 'cv2'
- Python was not found; run without arguments
- Attempted relative import with no known parent package
- TypeError: only integer scalar arrays can be converted to a scalar index
- A value is trying to be set on a copy of a slice from a DataFrame
- ValueError: setting an array element with a sequence
- Indentationerror: unindent does not match any outer indentation level
- Valueerror: if using all scalar values, you must pass an index
- ImportError: libGL.so.1: cannot open shared object file: No such file or directory
- Custom Exceptions in Python with Examples
- Python String replace() Method
- sqrt Python | Find the Square Root in Python
- Read JSON file using Python
- Binary search in Python
- Defaultdict in Python
- Int Object is Not Iterable – Python Error
- os.path.join in Python
- TypeError: int object is not subscriptable
- Python multiline comment
- Typeerror: str object is not callable
- Python reverse List
- zip() in Python for Parallel Iteration
- strftime() in Python
- Typeerror: int object is not callable
- Python List pop() Method
- Fibonacci series in Python
- Python any() function
- Python any() Vs all()
- Python pass Statement
- Python Lowercase - String lower() Method
- Modulenotfounderror: no module named istutils.cmd
- Append to dictionary in Python : Key/Value Pair
- timeit | Measure execution time of small code
- Python Decimal to Binary
- GET and POST requests using Python
- Difference between List VS Set in Python
- How to Build Word Cloud in Python?
- Binary to Decimal in Python
- Modulenotfounderror: no module named 'apt_pkg'
- Convert List to Array Python