Cannot convert float NaN to integer
The error "Cannot convert float NaN to integer" occurs when you attempt to explicitly convert a floating-point NaN (Not-a-Number) value to an integer. In Python, NaN is a special value representing undefined or missing data, and converting it to an integer is not possible due to the lack of a valid integer representation for NaN.
Direct Conversion of NaN to Integer
In this example, we attempt to convert the NaN value to an integer using the int() function. However, Python raises a ValueError because converting NaN to an integer is not possible.
Arithmetic Operation Involving NaN
In this example, we try to perform an addition operation between NaN and a valid integer. However, Python raises a TypeError because arithmetic operations involving NaN may lead to undefined results.
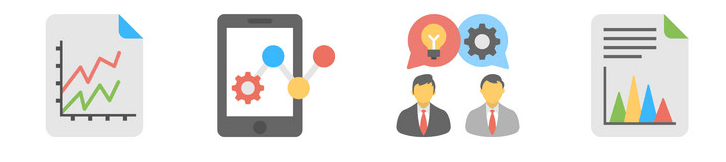
Using numpy.nan_to_num()
The numpy.nan_to_num() returns an array or scalar replacing Not a Number ( Not A Number ) with zero, positive_infinity with a very large number and negative_infinity with a very small (or negative) number.
Here you get the output value is NAN .
Next you can check the NAN value using isnan(value) , if it is NAN value then you can convert using nan_to_num() .
Here you can see the nan_to_num() changed the NaN value to 0.0 which can then be converted into an integer.
Full SourceAlso, consider the following steps:
- Avoid explicit conversions of NaN to integers. Instead, focus on handling missing or undefined data appropriately without attempting to convert NaN to integers.
- Use functions like np.isnan() from NumPy or math.isnan() from the math module to check for NaN values before performing operations or conversions.
- If needed, use functions like fillna() from Pandas or NumPy to replace NaN values with valid integers or other suitable default values, depending on your data processing requirements.
Conclusion
The error "Cannot convert float NaN to integer" occurs when you attempt to convert a floating-point NaN (Not-a-Number) value to an integer. Since NaN represents an undefined or missing value, it cannot be directly converted to an integer, as integers do not have a representation for NaN. This error often arises when performing arithmetic operations or type conversions involving NaN values. To handle this error, you can use appropriate checks or functions to handle missing data or convert NaN values to a valid integer representation, such as using the fillna() method to replace NaN with a default integer value.
- ImportError: No module named pandas
- What is SettingWithCopyWarning?
- UnicodeDecodeError while reading CSV file
- How to fix CParserError: Error tokenizing data
- ValueError: cannot reindex from a duplicate axis
- How to fix "Unnamed: 0" column in a pandas DataFrame
- ValueError: Unknown label type: 'unknown'
- ValueError: Length of values does not match length of index
- ValueError: The truth value of an array with more than..
- Attributeerror: 'dataframe' object has no attribute 'concat'