What Is Inheritance ?
Inheritance is indeed a foundational feature in Object-Oriented Programming. It involves the creation of a new class, known as the Derived Class, based on an existing class, referred to as the Base Class. This mechanism enables the reuse and modification of data and functionality already defined in the Base Class, while also allowing the addition of new data and functionality specific to the Derived Class.
Inheritance provides an elegant approach to building upon existing code, promoting code reusability and modularity. By inheriting from a Base Class, the Derived Class inherits all the properties and methods defined in the Base Class, thus expanding its set of available properties. This inheritance relationship establishes an "is-a" association, where the Derived Class can be viewed as a specialized version of the Base Class.
While inheriting properties from the Base Class, the Derived Class also has the flexibility to override or modify certain properties or methods inherited from the Base Class. This allows customization and adaptation of inherited behavior to suit the specific requirements of the Derived Class. By selectively overriding properties or methods, the Derived Class can introduce new or different functionality while still benefiting from the shared attributes and behaviors inherited from the Base Class.
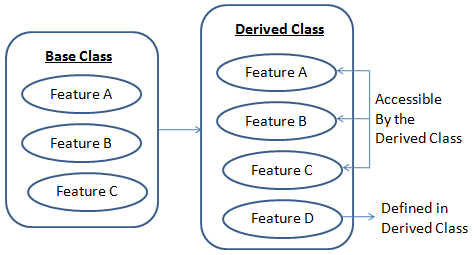
Inheritance and the .NET Framework
Inheritance offers numerous advantages, with code reusability being a key benefit. Instead of starting from scratch, developers can utilize existing code and build upon it, incorporating only the necessary new features. This approach significantly saves time and effort in software development. When a class is derived from another class, the derived class automatically inherits all the data and functionality of the base class, except for its constructors and destructors.
By utilizing inheritance, developers can avoid reinventing the wheel and tap into the capabilities already implemented in the base class. The derived class inherits the properties, methods, and behaviors defined in the base class, reducing the need to duplicate code and promoting a more streamlined and efficient development process.
The reuse of existing classes through inheritance also leads to better code organization and maintenance. It allows for modular development, where related classes can be grouped together based on their inheritance hierarchy. This promotes code encapsulation and separation of concerns, making the codebase more manageable and easier to maintain.
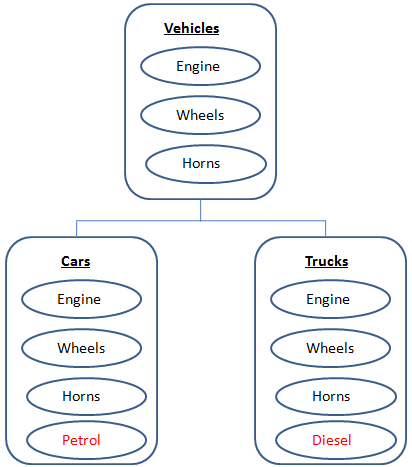
From the above example, Cars and Trucks have certain common properties; all have engine, wheels, horns etc. Thus they can be grouped under a Class called Vehicles. Apart from sharing these common features, each Derived Class has its own particular features - Cars use petrol while Trucks use diesel.
Multiple inheritance in .Net Framework
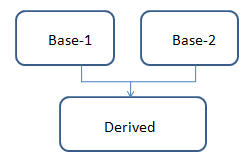
Multiple inheritance is a feature in some programming languages that allows a class to inherit properties and behaviors from multiple parent classes. However, in the .NET Framework, the concept of multiple inheritance is not directly supported. In .NET, a class is only permitted to inherit from a single parent class, which is referred to as single inheritance.
Single inheritance promotes a simpler and more structured class hierarchy. It ensures that each class has a clear and unambiguous parent, avoiding conflicts and complications that may arise from inheriting from multiple classes with potentially conflicting properties and behaviors.
The .NET Framework offers alternative mechanisms to achieve similar functionalities as multiple inheritance. One approach is through interface implementation. Interfaces define contracts that specify a set of methods and properties that a class must implement. By implementing multiple interfaces, a class can exhibit behaviors and capabilities from multiple sources, effectively achieving a form of multiple inheritance in terms of functionality.
Another approach is through composition, where a class can contain instances of other classes as member objects, thereby incorporating their functionalities. This allows for combining different classes and their functionalities without directly inheriting from multiple parent classes.
While multiple inheritance offers flexibility in certain scenarios, single inheritance, along with interface implementation and composition, ensures better code organization, reduces complexities, and maintains a more manageable class hierarchy in the .NET Framework.
However, inheritance may be implemented in different combinations in Object-Oriented Programming languages as illustrated in figure and they include:
- Single Inheritance
- Multi Level Inheritance
- Hierarchical Inheritance
- Hybrid Inheritance
- Multipath inheritance
- Multiple Inheritance
Conclusion
Inheritance in Object-Oriented Programming provides a powerful means to reuse, extend, and modify existing code. It enables the creation of Derived Classes that inherit properties and methods from a Base Class while offering the ability to override or introduce new functionalities, promoting code organization, and facilitating code maintenance and scalability.
- What is object-oriented programming?
- What is a Class?
- What is an Object?
- Constructors and Destructors
- What are the different types of inheritance ?
- What are Access Modifiers ?
- Why Classes cannot be declared as Protected?
- Can we declare private class in namespace
- Difference between Classes and Structures
- Can we use pointers in C# ?
- Why abstract class can't create instance
- Can you prevent your class from being inherited
- Difference between method Overloading and Overriding
- Difference between Early Binding and Late binding
- What is nested class
- What is partial class ?
- What is Virtual Method
- Difference between class and object
- What is Data Encapsulation?
- Object Based Language and OOPs
- SOLID Principles in C#
- Solid Principles | Advantages and Disadvantages