What is nested class
A nested class, also known as an inner class, is a class that is defined within another class. It exists within the scope of the outer class and can access the members of the outer class, including private members. Nested classes provide a way to logically group related classes and can enhance code organization and encapsulation. There are four types of nested classes in C#: nested class, nested struct, nested interface, and nested delegate. Let's explore each type with examples:
Nested Class
A nested class is a class declared inside another class. It can access all members (including private members) of the outer class. It is often used to encapsulate related functionality within the outer class.
In the above example, the NestedClass is declared within the OuterClass. It can access the private field outerField of the outer class directly.
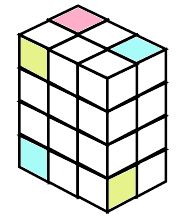
Nested classes are useful when you want to group related classes together or when the nested class needs access to the private members of the outer class.
Nested Struct
Similar to nested classes, nested structs are structs defined within another class. They have the same accessibility and scoping rules as nested classes.
In the above example, the NestedStruct is defined within the OuterClass. It can be used just like any other struct, but its scope is limited to the OuterClass.
Nested Interface
A nested interface is an interface declared within another class or interface. It is typically used to define a specialized interface related to the enclosing class or interface.
In the above example, the INestedInterface is a nested interface declared within the OuterClass. It can be implemented by any class or struct.
Nested Delegate
A nested delegate is a delegate declared within another class or struct. It provides a way to define a delegate type within a specific context.
In the above example, the NestedDelegate is a nested delegate declared within the OuterClass. It can be used to pass methods as arguments to the UseDelegate method.
Conclusion
Nested classes can improve code organization, encapsulation, and logical grouping of related classes within a larger class. They provide access to the members of the outer class and allow for more structured and modular code design.
- What is object-oriented programming?
- What is a Class?
- What is an Object?
- Constructors and Destructors
- What Is Inheritance ?
- What are the different types of inheritance ?
- What are Access Modifiers ?
- Why Classes cannot be declared as Protected?
- Can we declare private class in namespace
- Difference between Classes and Structures
- Can we use pointers in C# ?
- Why abstract class can't create instance
- Can you prevent your class from being inherited
- Difference between method Overloading and Overriding
- Difference between Early Binding and Late binding
- What is partial class ?
- What is Virtual Method
- Difference between class and object
- What is Data Encapsulation?
- Object Based Language and OOPs
- SOLID Principles in C#
- Solid Principles | Advantages and Disadvantages