What is partial class ?
A partial class in C# is a class that is split into multiple files. Each file contains a portion of the class definition, and all parts combined create a single class. The partial class feature allows developers to divide a class's implementation across multiple files, making it easier to manage and maintain large classes or auto-generated code.
Syntax:To define a partial class, you use the partial keyword in the class declaration. Each part of the class must have the same name and be marked as partial.
Example of a partial class:In the example above, the MyClass is split into two files (MyClass1.cs and MyClass2.cs) using the partial keyword.
Combining Parts
During compilation, the compiler merges all the partial class parts into a single class. The resulting class has the combined members and methods from all the partial class files.
Accessibility and Inheritance
All parts of a partial class must have the same accessibility level. If any part is marked as public, the entire class is considered public. Similarly, if any part is marked as private, the entire class is considered private.
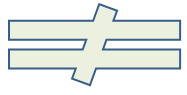
Partial classes can also participate in inheritance. In other words, you can derive a class from a partial class, and additional parts of the derived class can be defined in separate files.
Purpose
Partial classes are primarily used in scenarios such as code generation, tool-generated code, or when multiple developers need to work on different parts of a large class simultaneously. It allows for separation of concerns, better organization, and easier maintenance of complex classes.
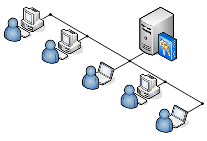
For example, if you are using a visual designer or code generator to create a Windows Form, the generated code is often placed in a partial class. You can then add custom code to another part of the partial class without modifying the generated code directly.
Partial classes should be used with caution, as they can lead to code fragmentation and confusion if misused. It's important to maintain proper organization and adhere to good coding practices when using partial classes.
Conclusion
Partial classes in C# provide a way to split a class definition across multiple files while maintaining a cohesive single class at compile-time.
- What is object-oriented programming?
- What is a Class?
- What is an Object?
- Constructors and Destructors
- What Is Inheritance ?
- What are the different types of inheritance ?
- What are Access Modifiers ?
- Why Classes cannot be declared as Protected?
- Can we declare private class in namespace
- Difference between Classes and Structures
- Can we use pointers in C# ?
- Why abstract class can't create instance
- Can you prevent your class from being inherited
- Difference between method Overloading and Overriding
- Difference between Early Binding and Late binding
- What is nested class
- What is Virtual Method
- Difference between class and object
- What is Data Encapsulation?
- Object Based Language and OOPs
- SOLID Principles in C#
- Solid Principles | Advantages and Disadvantages