How to Prevent Inheritance
Inheritance in .NET allows one class (the derived class) to inherit the members of another class (the base class). However, there may be scenarios where you want to prevent a class from being inherited, effectively disabling inheritance for that class. In .NET, you can achieve this by using the sealed keyword.
The sealed keyword is used to specify that a class or a class member cannot be inherited or overridden by other classes. Here's how you can prevent inheritance using the sealed keyword in .NET:
Preventing Inheritance for a Class
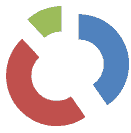
In the example above, the SealedClass is declared with the sealed keyword. This means that other classes cannot inherit from SealedClass, and any attempt to do so will result in a compilation error.
Preventing Inheritance for Class Members
In the example above, the SealedMethod() is declared with the sealed keyword. This means that any derived class cannot override this method. If a derived class attempts to override it, a compilation error will occur.
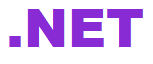
Preventing inheritance using the sealed keyword ensures that the class or class member cannot be extended or modified, maintaining its intended behavior. It's worth noting that the sealed keyword can only be used with classes that are declared as public, as it doesn't make sense to seal a class that is not accessible from other code.
Here's an example to demonstrate how inheritance prevention works:
In the above example, we attempt to derive a class named DerivedClass from the SealedClass, which is declared as sealed. As a result, a compilation error occurs, preventing the inheritance.
- What is object-oriented programming?
- What is a Class?
- What is an Object?
- Constructors and Destructors
- What Is Inheritance ?
- What are the different types of inheritance ?
- What are Access Modifiers ?
- Why Classes cannot be declared as Protected?
- Can we declare private class in namespace
- Difference between Classes and Structures
- Can we use pointers in C# ?
- Why abstract class can't create instance
- Difference between method Overloading and Overriding
- Difference between Early Binding and Late binding
- What is nested class
- What is partial class ?
- What is Virtual Method
- Difference between class and object
- What is Data Encapsulation?
- Object Based Language and OOPs
- SOLID Principles in C#
- Solid Principles | Advantages and Disadvantages