How to convert string to boolean | JavaScript
In JavaScript, you can convert a string to a boolean using a few different approaches. Here are the details along with examples:
Using Boolean Constructor
You can use the Boolean constructor to explicitly convert a string to a boolean value. It converts an empty string to false and any non-empty string to true.
Keep in mind that all non-empty strings are converted to true, including strings like "false".
Using Comparison
You can perform a comparison to achieve implicit string-to-boolean conversion. Any non-empty string, including "false", will be evaluated as true, while an empty string will be evaluated as false.
Using JSON.parse()
This method can be used if the input string follows JSON syntax. JSON.parse() will correctly convert the string to its boolean representation.
Remember that these conversions are sensitive to the exact content of the string. For best results, use consistent and expected values.
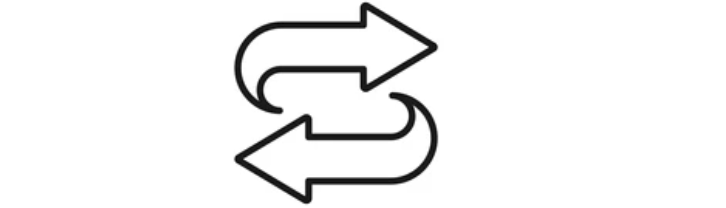
Using Regular Expression
The test() method of JavaScript matches a regular expression against a string.
The "/i" at the end of regular expression is for case insensitive match.
Note: If the string contains something other than "true" or "false", these methods might not give you the desired boolean result and might instead produce false.
Conclusion
You can convert a string to a boolean using methods like the Boolean constructor or comparisons for implicit conversion. The Boolean constructor converts non-empty strings to true and empty strings to false, while comparisons evaluate non-empty strings as true and empty strings as false. Additionally, JSON.parse() can be used for accurate conversion if the string follows JSON syntax.
- JavaScript Popup Boxes
- Opening a new window in JavaScript
- How to Create Drop-Down Lists in JavaScript
- How do I include a JavaScript file in another JavaScript file?
- Print the content of a Div using JavaScript/jQuery
- How to get the current URL using JavaScript ?
- How to Detect a Mobile Device with JavaScript/jQuery
- How to validate an email address in JavaScript
- JavaScript Array Iteration
- How to Remove a Specific Item from an Array in JavaScript
- What is JavaScript closures?
- How To Remove a Property from a JavaScript Object
- How to get selected value from Dropdown list in JavaScript
- How do I get the current date in JavaScript?
- How to Open URL in New Tab | JavaScript
- How to delay/wait/Sleep in code execution | JavaScript
- How to round to at most 2 decimal places | JavaScript
- How to check undefined in JavaScript?
- How To Copy to Clipboard | JavaScript
- How to encode a URL using JavaScript?
- How to force Input field to enter numbers only | JavaScript
- How to create multiline string in JavaScript
- How to Check for an Empty String in JavaScript?