How to get current URL with JavaScript?
JavaScript offers multiple methods to interact with the current URL displayed in the web browser's address bar. These methods are facilitated through the Location object, which is a property of the Window object. You can utilize the Location object's properties to acquire information about the current page's address or employ its methods to perform actions like redirecting or refreshing the page. This provides developers with a versatile toolkit for managing and interacting with the browser's URL.

URL of this page is:
URL structure
URL's are also called links. It is an address on the internet. It's made up of a protocol , domain name, and a path.
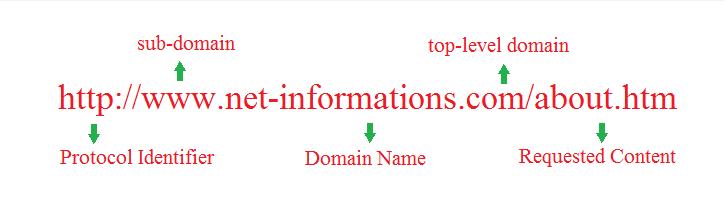
JavaScript window.location properties
Using these window.location object properties you can access all of these URL components and what they can set or return:
- href - Full URL (http://www.example.com:8082/index.php#tab2)
- protocol - Protocol Schema (http: or https)
- host - Domain name + port (www.example.com:8082)
- hostname - Domain name (www.example.com)
- port - Port Number (8082)
- pathname - the path name of the URL (index.php)
- search - ? followed by Query String (?foo=789)
- hash - # followed by the Anchor or Fragment identifier (#tab2)

window.location properties in javascript
Conclusion
To retrieve the current URL using JavaScript, utilize the window.location.href property. This provides a straightforward way to access and utilize the URL of the current webpage in your scripts.
- JavaScript Popup Boxes
- Opening a new window in JavaScript
- How to Create Drop-Down Lists in JavaScript
- How do I include a JavaScript file in another JavaScript file?
- Print the content of a Div using JavaScript/jQuery
- How to Detect a Mobile Device with JavaScript/jQuery
- How to validate an email address in JavaScript
- JavaScript Array Iteration
- How to Remove a Specific Item from an Array in JavaScript
- What is JavaScript closures?
- How To Remove a Property from a JavaScript Object
- How to get selected value from Dropdown list in JavaScript
- How do I get the current date in JavaScript?
- How to Open URL in New Tab | JavaScript
- How to delay/wait/Sleep in code execution | JavaScript
- How to round to at most 2 decimal places | JavaScript
- How to convert string to boolean | JavaScript
- How to check undefined in JavaScript?
- How To Copy to Clipboard | JavaScript
- How to encode a URL using JavaScript?
- How to force Input field to enter numbers only | JavaScript
- How to create multiline string in JavaScript
- How to Check for an Empty String in JavaScript?