How to set time delay in JavaScript
JavaScript's nature as a scripting language and the concept of synchronous, single-threaded execution. While it's generally discouraged to introduce blocking delays in the execution flow, there are legitimate scenarios where controlled time delays are needed to achieve specific behaviors. In such cases, JavaScript provides various functions and methods to facilitate these timed actions.
These functions allow developers to perform actions, introduce pauses, and subsequently carry out further actions, enhancing the interactive behavior of JavaScript applications. It's important to use these mechanisms thoughtfully and avoid introducing unnecessary blocking delays in the execution flow.
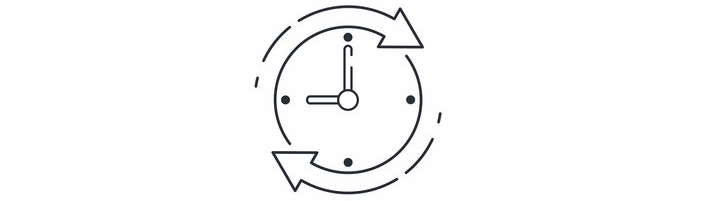
Setting a time delay in JavaScript can be achieved using the setTimeout() and setInterval() functions. These functions allow you to schedule the execution of code after a certain amount of time. Here's a detailed explanation along with examples for both methods:
Javascript setTimeout()
The first and the most common method in implementing a delay in the execution of a JavaScript code is to use the setTimeOut() method.
- functionname - The function name for the function to be executed.
- milliseconds - The number of milliseconds.

Javascript setInterval()
JavaScript's setTimeout() executes only once after the specified delay, whereas setInterval() repeatedly calls the callback function at regular intervals. Both of these methods return an integer identifier that can be used to clear the scheduled execution before the timer expires. The setInterval() method continues calling the function until clearInterval() is invoked or the window is closed."
- functionname - The function name for the function to be executed.
- milliseconds - The number of milliseconds.

Javascript sleep()
JavaScript has undergone substantial evolution, enabling the simulation of the sleep() function through various approaches. The introduction of features like promises and async/await functions in JavaScript has greatly facilitated the utilization of the sleep() function, streamlining its implementation. These advancements offer more convenient ways to introduce delays and manage asynchronous behavior in JavaScript code.
Or as a one-liner:
Or
The sleep() function can be used along with the async/await to get the pause between the execution.
exampleClearing Timeout or Interval
To clear a timeout or interval, you can use the clearTimeout() and clearInterval() functions, respectively. These functions require the identifier returned by the original setTimeout() or setInterval() call.
Conclusion
To introduce time delays in JavaScript, you can use the setTimeout() function for a one-time delay or the setInterval() function for repeated delays. These functions enable you to execute code after a specified time interval, enhancing the interactivity and behavior of your web applications. Remember to clear timeouts or intervals when necessary using clearTimeout() and clearInterval() to prevent unwanted execution.
- JavaScript Popup Boxes
- Opening a new window in JavaScript
- How to Create Drop-Down Lists in JavaScript
- How do I include a JavaScript file in another JavaScript file?
- Print the content of a Div using JavaScript/jQuery
- How to get the current URL using JavaScript ?
- How to Detect a Mobile Device with JavaScript/jQuery
- How to validate an email address in JavaScript
- JavaScript Array Iteration
- How to Remove a Specific Item from an Array in JavaScript
- What is JavaScript closures?
- How To Remove a Property from a JavaScript Object
- How to get selected value from Dropdown list in JavaScript
- How do I get the current date in JavaScript?
- How to Open URL in New Tab | JavaScript
- How to round to at most 2 decimal places | JavaScript
- How to convert string to boolean | JavaScript
- How to check undefined in JavaScript?
- How To Copy to Clipboard | JavaScript
- How to encode a URL using JavaScript?
- How to force Input field to enter numbers only | JavaScript
- How to create multiline string in JavaScript
- How to Check for an Empty String in JavaScript?