How to copy text to the Clipboard | JavaScript
Copying text to the clipboard in JavaScript involves interacting with the Clipboard API. Here are the details along with examples:
Using the Clipboard API (Async Clipboard API)
The modern and recommended way is to use the Clipboard API, which is asynchronous and provides better control over clipboard interactions.
Fallback Approach (for browsers without Clipboard API)
If the Clipboard API isn't supported, you can provide a fallback method using the document.execCommand() approach mentioned earlier.
Using the Document.execCommand() Method (Deprecated)
The execCommand() method, although deprecated, is still widely used to copy text to the clipboard. It works in most browsers but may not be supported in the future.
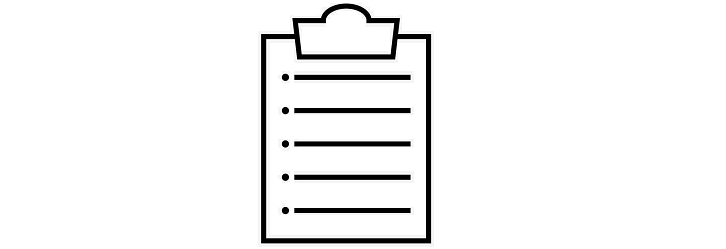
Clipboard API
The Clipboard interface represents the Clipboard API, allowing web applications to request both read and write access to the system clipboard, subject to user permissions. The navigator.clipboard API is a more recent addition to the web specification, enabling easier clipboard manipulation, but its support might not be consistent across all browsers. Developers should consider providing fallback solutions for browsers that do not fully implement the navigator.clipboard API.
Conclusion
The Clipboard API offers better security and user control, and it's the recommended way to copy text to the clipboard in modern browsers. However, in some cases, you might need to provide a fallback mechanism for older browsers that don't support the API.
- JavaScript Popup Boxes
- Opening a new window in JavaScript
- How to Create Drop-Down Lists in JavaScript
- How do I include a JavaScript file in another JavaScript file?
- Print the content of a Div using JavaScript/jQuery
- How to get the current URL using JavaScript ?
- How to Detect a Mobile Device with JavaScript/jQuery
- How to validate an email address in JavaScript
- JavaScript Array Iteration
- How to Remove a Specific Item from an Array in JavaScript
- What is JavaScript closures?
- How To Remove a Property from a JavaScript Object
- How to get selected value from Dropdown list in JavaScript
- How do I get the current date in JavaScript?
- How to Open URL in New Tab | JavaScript
- How to delay/wait/Sleep in code execution | JavaScript
- How to round to at most 2 decimal places | JavaScript
- How to convert string to boolean | JavaScript
- How to check undefined in JavaScript?
- How to encode a URL using JavaScript?
- How to force Input field to enter numbers only | JavaScript
- How to create multiline string in JavaScript
- How to Check for an Empty String in JavaScript?