Remove a property from JavaScript object
Removing a property from a JavaScript object can be accomplished using various methods. Let's explore these methods with detailed explanations and examples:
Using the delete Keyword
The delete keyword can be used to remove a property from an object.
Using Object Destructuring
Object destructuring can be used to create a new object without the property to be removed.
Using Object.assign()
Object.assign() can be utilized to create a new object without the property to be removed.
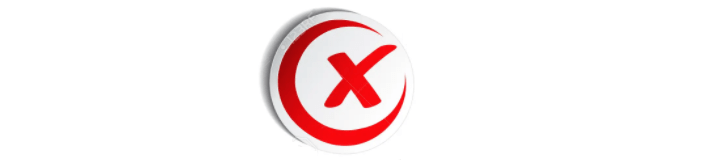
Using the Spread Operator
The spread operator (...) can also be used to create a new object without the property.
Using Object.keys() and Object.fromEntries()
You can also use Object.keys() and Object.fromEntries() to create a new object without the property.
Conclusion
Removing a property from a JavaScript object can be achieved using methods like the delete keyword, object destructuring, Object.assign(), the spread operator, and a combination of Object.keys() and Object.fromEntries(). The method you choose depends on your coding style and specific use case.
- JavaScript Popup Boxes
- Opening a new window in JavaScript
- How to Create Drop-Down Lists in JavaScript
- How do I include a JavaScript file in another JavaScript file?
- Print the content of a Div using JavaScript/jQuery
- How to get the current URL using JavaScript ?
- How to Detect a Mobile Device with JavaScript/jQuery
- How to validate an email address in JavaScript
- JavaScript Array Iteration
- How to Remove a Specific Item from an Array in JavaScript
- What is JavaScript closures?
- How to get selected value from Dropdown list in JavaScript
- How do I get the current date in JavaScript?
- How to Open URL in New Tab | JavaScript
- How to delay/wait/Sleep in code execution | JavaScript
- How to round to at most 2 decimal places | JavaScript
- How to convert string to boolean | JavaScript
- How to check undefined in JavaScript?
- How To Copy to Clipboard | JavaScript
- How to encode a URL using JavaScript?
- How to force Input field to enter numbers only | JavaScript
- How to create multiline string in JavaScript
- How to Check for an Empty String in JavaScript?