Round a Number to 2 Decimal Places | JavaScript
You can use:
But, it seems like Math.round() is a better solution, but it is not! In some cases it will NOT round correctly. Also, toFixed() will NOT round correctly in some cases.
Approximate Rounding
To address rounding issues associated with Math.round() and toFixed(), you can create a custom rounding function that incorporates a "nearly equal" test. This test helps decide whether a fractional value is close enough to a midpoint value to warrant midpoint rounding adjustments. By using this method, you can achieve more accurate rounding results.
The following function return the value of the given number rounded to the nearest integer accurately.
When using a custom rounding function that incorporates a "nearly equal" test for halfway numbers (numbers that are equidistant between two possible rounded values), you might still occasionally encounter scenarios where the result is the lower rounded value due to the inherent limitations of floating-point arithmetic. This behavior is a result of how computers represent and manipulate numbers with finite precision. While the custom rounding function improves accuracy in many cases, it's essential to be aware that rounding can still produce unexpected outcomes for certain inputs.
Using Number.toLocaleString()
The toLocaleString() method in JavaScript is primarily designed for formatting numbers according to the user's locale, including adding thousands separators and using the appropriate decimal separator. However, it doesn't inherently provide accurate rounding functionality.
While you mentioned a custom rounding function using Number.toLocaleString() for accurate rounding to the nearest integer, it's important to clarify that toLocaleString() itself doesn't perform rounding operations in the way other rounding methods do. If you could provide the details or code of the custom function you're referring to, I'd be happy to help clarify its behavior and accuracy.
Rounding
Rounding involves approximating a number while maintaining its value close to the original. There isn't a one-size-fits-all solution applicable to everyone. Various rounding algorithms exist, and your implementation might differ based on your specific requirements.
Rounding Numbers in JavaScript
In many instances, JavaScript developers employ Math.round() or toFixed() to round numbers to the nearest integer. However, while Math.round() might appear superior, it isn't infallible. There are situations where it fails to round accurately. Similarly, toFixed() also exhibits shortcomings and doesn't consistently achieve correct rounding in certain scenarios.
Math.round()
In the above case the output is 5.01 instead of 5.02. If you execute the 5.015 with the above function roundTo() the output will be 5.02, that is the correct answer.
toFixed()
In the above case the output is 1.55 instead of 1.56 . If you execute the 1.555 with the above function roundTo() the output will be 1.56, that is the correct answer.
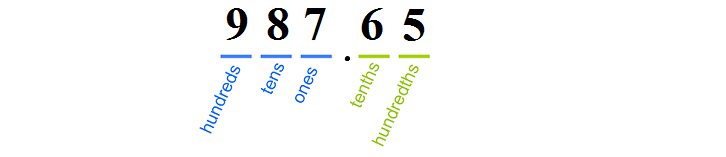
When the rounded number is larger than the original, the given number is considered rounded up. Conversely, if the rounded number is smaller, it is considered rounded down.
Conclusion
To round a number to two decimal places in JavaScript, you can use the toFixed() method on a number to directly obtain a string representation with the desired precision. Alternatively, you can use Math.round() in combination with multiplication and division to achieve the same result. Remember that rounding can introduce minor precision discrepancies due to the nature of floating-point arithmetic.
- JavaScript Popup Boxes
- Opening a new window in JavaScript
- How to Create Drop-Down Lists in JavaScript
- How do I include a JavaScript file in another JavaScript file?
- Print the content of a Div using JavaScript/jQuery
- How to get the current URL using JavaScript ?
- How to Detect a Mobile Device with JavaScript/jQuery
- How to validate an email address in JavaScript
- JavaScript Array Iteration
- How to Remove a Specific Item from an Array in JavaScript
- What is JavaScript closures?
- How To Remove a Property from a JavaScript Object
- How to get selected value from Dropdown list in JavaScript
- How do I get the current date in JavaScript?
- How to Open URL in New Tab | JavaScript
- How to delay/wait/Sleep in code execution | JavaScript
- How to convert string to boolean | JavaScript
- How to check undefined in JavaScript?
- How To Copy to Clipboard | JavaScript
- How to encode a URL using JavaScript?
- How to force Input field to enter numbers only | JavaScript
- How to create multiline string in JavaScript
- How to Check for an Empty String in JavaScript?