Open a URL in a new tab (and not a new window)
The behavior of opening a URL in a new tab or window is ultimately determined by the user's browser preferences and settings. JavaScript, through the window.open() method, doesn't have the power to override these preferences. The method can request a new window or tab based on its parameters, but the final decision rests with the browser and its settings. Users typically have the freedom to choose whether links open in a new tab or window based on their browsing preferences.
Open URL in New Tab using JavaScript
When using the window.open() method in JavaScript to open a URL in a new tab, using "_blank" as the second parameter is the correct approach. This parameter value instructs the browser to open the URL in a new tab.
Additionally, you're right that you should avoid adding a third parameter to the window.open() method. Omitting the third parameter ensures that the behavior adheres to opening the URL in a new tab as intended, instead of potentially opening a new window.

Click the button to open a new tab
You could do it this way calling a direct function, or by adding an event listener to your DOM object.
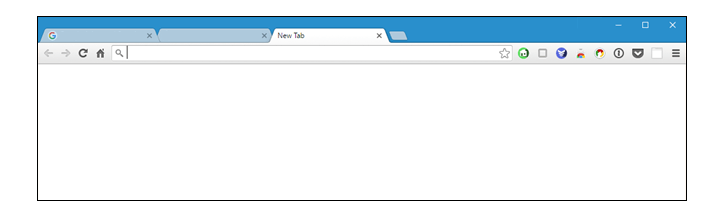
Method-2 : Open URL in New Tab

Click the button to open a new tab
The behavior of the window.open() method, particularly in terms of opening URLs in new tabs or windows, can vary across different web browsers like Internet Explorer, Opera, Firefox, and Chrome. This variation arises from the fact that browsers may interpret user preferences and settings differently, leading to different outcomes when the method is used.
As a result, web developers should be aware that while using window.open() with "_blank" can generally open URLs in new tabs, the actual behavior may not be consistent across all browsers due to their individual handling of user preferences. It's always a good practice to test and ensure the desired behavior across various browsers.
Conclusion
To open a URL in a new tab using JavaScript, utilize the window.open() method with the "_blank" parameter. This approach offers a simple way to provide users with quick access to external resources without navigating away from your website.
- JavaScript Popup Boxes
- Opening a new window in JavaScript
- How to Create Drop-Down Lists in JavaScript
- How do I include a JavaScript file in another JavaScript file?
- Print the content of a Div using JavaScript/jQuery
- How to get the current URL using JavaScript ?
- How to Detect a Mobile Device with JavaScript/jQuery
- How to validate an email address in JavaScript
- JavaScript Array Iteration
- How to Remove a Specific Item from an Array in JavaScript
- What is JavaScript closures?
- How To Remove a Property from a JavaScript Object
- How to get selected value from Dropdown list in JavaScript
- How do I get the current date in JavaScript?
- How to delay/wait/Sleep in code execution | JavaScript
- How to round to at most 2 decimal places | JavaScript
- How to convert string to boolean | JavaScript
- How to check undefined in JavaScript?
- How To Copy to Clipboard | JavaScript
- How to encode a URL using JavaScript?
- How to force Input field to enter numbers only | JavaScript
- How to create multiline string in JavaScript
- How to Check for an Empty String in JavaScript?