Can we create the instance for abstract classes
No, you cannot create an instance of an abstract class because it serves as an incomplete or partially implemented class. The main purpose of an abstract class is to serve as a base for derived subclasses to inherit from and build upon. It provides a blueprint or template for the derived classes to follow and implement the missing functionality.
Abstract class
An abstract class contains one or more abstract methods, which are methods without any implementation details. These abstract methods are meant to be overridden and implemented by the derived classes. The abstract class may also contain non-abstract methods with complete implementations that can be inherited directly by the derived classes.
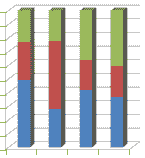
By declaring a class as abstract, you are signaling that it is not intended to be instantiated directly. Instead, it provides a foundation for more specialized classes to extend and implement the missing functionality. The derived classes that inherit from the abstract class must provide concrete implementations for the abstract methods to make the class complete and usable.
Here's an example that demonstrates the use of an abstract class:
In this example, the Shape class is declared as abstract with an abstract method CalculateArea(). The Circle and Rectangle classes inherit from the Shape class and provide concrete implementations for the CalculateArea() method. You can create instances of Circle and Rectangle objects and invoke their respective CalculateArea() and Display() methods.
Conclusion
Abstract classes are useful when you want to define common behavior and characteristics among a group of related classes, while allowing each subclass to provide its own specific implementation. They promote code reusability and enforce a consistent structure within the inheritance hierarchy.
- What is object-oriented programming?
- What is a Class?
- What is an Object?
- Constructors and Destructors
- What Is Inheritance ?
- What are the different types of inheritance ?
- What are Access Modifiers ?
- Why Classes cannot be declared as Protected?
- Can we declare private class in namespace
- Difference between Classes and Structures
- Can we use pointers in C# ?
- Can you prevent your class from being inherited
- Difference between method Overloading and Overriding
- Difference between Early Binding and Late binding
- What is nested class
- What is partial class ?
- What is Virtual Method
- Difference between class and object
- What is Data Encapsulation?
- Object Based Language and OOPs
- SOLID Principles in C#
- Solid Principles | Advantages and Disadvantages