What is a Class?
A class in object-oriented programming is a fundamental building block that serves as a blueprint or template for creating objects. It defines the structure, behavior, and attributes that objects of that class will possess. A class encapsulates data, known as member variables or properties, and the methods or functions that operate on that data. It acts as a container for organizing related data and functions into a cohesive unit.
Common characteristics and behaviors
A class specifies the common characteristics and behaviors that its objects will exhibit. It describes the properties or attributes that objects will have, allowing them to store and manage data. Additionally, a class defines the methods or functions that enable objects to perform specific actions or operations. These methods can manipulate the internal state of an object and interact with other objects.
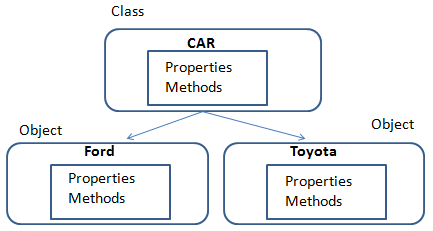
In everyday life, objects with similar characteristics can be categorized and grouped together based on specific criteria. For instance, if we consider a Ford car and a Toyota car, both fall under the broader category of Cars. As a result, they can be classified as belonging to the Car class. In fact, there may be numerous other cars in existence, all sharing the same make and model. Each individual car is constructed using the same set of blueprints, thus possessing identical components and attributes.
In the scope of object-oriented programming, we can draw parallels to this real-life scenario. We can regard your personal car as an object or instance of the class named CAR. The CAR class serves as a template that outlines the structure, attributes, and behaviors that all car objects should possess. By utilizing this class template, you can create multiple instances of cars, each representing a distinct object. The class acts as a blueprint, while the objects themselves are concrete manifestations based on that template.
This concept of classes and objects allows for flexibility and reusability in programming. It enables the creation of various objects that adhere to the same class structure, while allowing each object to have its specific data and behavior. By employing classes and objects, object-oriented programming facilitates modularity, encapsulation, and code organization, much like how real-world objects can be grouped based on common characteristics.
Object Oriented Programming representation of a Car Class
Conclusion
A class acts as a blueprint or template that defines the structure and behavior of objects. It provides a means to create multiple instances of objects with consistent properties and behavior, supporting code reusability, modularity, and encapsulation within the field of object-oriented programming.
- What is object-oriented programming?
- What is an Object?
- Constructors and Destructors
- What Is Inheritance ?
- What are the different types of inheritance ?
- What are Access Modifiers ?
- Why Classes cannot be declared as Protected?
- Can we declare private class in namespace
- Difference between Classes and Structures
- Can we use pointers in C# ?
- Why abstract class can't create instance
- Can you prevent your class from being inherited
- Difference between method Overloading and Overriding
- Difference between Early Binding and Late binding
- What is nested class
- What is partial class ?
- What is Virtual Method
- Difference between class and object
- What is Data Encapsulation?
- Object Based Language and OOPs
- SOLID Principles in C#
- Solid Principles | Advantages and Disadvantages