Difference between class and object
In object-oriented programming, both classes and objects play fundamental roles. A class is a blueprint or template that defines the structure and behavior of objects, while an object is an instance of a class. Let's explore the differences between classes and objects in more detail with examples:
Class
- Definition: A class is a construct that defines a set of properties (attributes) and behaviors (methods) that an object of that class will possess. It acts as a blueprint or a template for creating objects.
- Usage: Classes provide a way to create objects with similar characteristics and functionalities. They encapsulate data and methods that operate on that data.
In the above example, the Car class defines properties like Model and Color and methods like StartEngine() and Accelerate(). It provides a blueprint for creating car objects.
Object
- Definition: An object is an instance of a class. It represents a specific entity or item that has attributes and behaviors defined by its class.
- Creation: Objects are created using the new keyword followed by the class name, which invokes the class's constructor to initialize the object.
- Usage: Objects encapsulate data and allow the execution of methods defined in their class. They can interact with other objects and be used to model real-world entities or represent abstract concepts.
In the above example, an object myCar is created using the Car class. The object has specific attributes (model: "Toyota Camry", color: "Blue") and can invoke methods like StartEngine() and Accelerate().
Key Differences between Class and Object
- Definition: A class is a blueprint or template that defines the structure and behavior of objects. An object is an instance of a class that represents a specific entity or item.
- Creation: Classes are defined in code, while objects are created at runtime by instantiating a class using the new keyword.
- Usage: Classes define properties and methods that objects possess. Objects encapsulate data and can invoke methods defined in their class.
- Relationship: A class describes the common properties and behaviors that objects of that class will have. Multiple objects can be created from a single class, each having its own state and behavior.
- Accessibility: Class members can have different access modifiers (public, private, protected) to control their visibility and accessibility. Objects provide access to the public members of their class.
Example
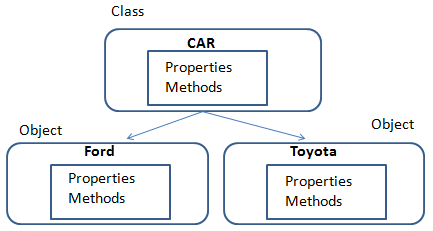
Here is an illustration that can help provide clarity on the above concepts. Let us consider a class named "CAR." In this class, all cars possess common attributes such as bodies, engines, and other pertinent features. These attributes can be defined as properties within our CAR class. Additionally, we can incorporate methods, which are essentially functions, that encompass functionalities shared by all cars, such as movement in both forward and reverse directions, since all cars are capable of moving. It is crucial to internalize the notion that the fundamental structure, or "template," of a car remains consistent. Each individual object is constructed based on this shared template (the class), and thus possesses the same constituent elements. All objects derive from the same set of member functions (methods), while maintaining distinct copies of member data (properties). For instance, both a Ford car and a Toyota car fall under the category of Cars, and can therefore be classified as belonging to the Car class. They share common movement capabilities (methods), but vary in terms of their specific models (properties).
Conclusion
A class defines the structure and behavior of objects, whereas an object is an instance of a class that represents a specific entity with its own state and behavior. Classes act as blueprints, and objects are the actual entities created based on those blueprints.
- What is object-oriented programming?
- What is a Class?
- What is an Object?
- Constructors and Destructors
- What Is Inheritance ?
- What are the different types of inheritance ?
- What are Access Modifiers ?
- Why Classes cannot be declared as Protected?
- Can we declare private class in namespace
- Difference between Classes and Structures
- Can we use pointers in C# ?
- Why abstract class can't create instance
- Can you prevent your class from being inherited
- Difference between method Overloading and Overriding
- Difference between Early Binding and Late binding
- What is nested class
- What is partial class ?
- What is Virtual Method
- What is Data Encapsulation?
- Object Based Language and OOPs
- SOLID Principles in C#
- Solid Principles | Advantages and Disadvantages