Constructors and Destructors
Constructors
A constructor is indeed a special member function within a class, sharing the same name as the class itself. It plays a vital role in object initialization within object-oriented languages. Each object created from a class possesses its own copy of member data, which requires proper initialization before it can be utilized. Constructors serve the purpose of automatically initializing objects as they are created, simplifying the initialization process.
The primary responsibility of a constructor is to ensure that the member variables of a class are correctly set to their initial values. By invoking the constructor, an object is instantiated with its member variables initialized to predefined values or values provided during object creation. Constructors enable the object to configure itself during the creation process, preparing it for usage without the need for separate initialization steps.
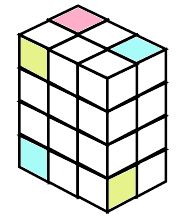
The presence of constructor methods streamlines the process of initializing class member variables, enhancing code readability and maintainability. They eliminate the burden of manually initializing object data, as the constructor takes care of this task automatically. This results in more efficient and organized code, facilitating the creation and proper initialization of objects within an object-oriented programming paradigm.
Declaration of Constructors
A constructor is optional, if no constructors are declared for a class, the compiler invokes a default constructor for you. The default constructor simply sets all the fields in the class to their default values. You can define as many constructors as you want, as long as each constructor has a different parameter list. Also not that constructor functions cannot return values.
Destructors
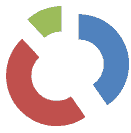
A destructor, denoted by a tilde (~) followed by the class name, is a special member function in object-oriented programming that serves to de-initialize objects when they are being destroyed. While constructors are responsible for initializing objects during creation, destructors play the opposite role by executing specific cleanup operations when objects are no longer needed.
When an object is destroyed, typically through the built-in garbage collection mechanism in many programming languages, the destructor is automatically invoked. The destructor's purpose is to release any resources acquired by the object during its lifetime, such as freeing memory allocations, closing open files, or releasing connections to external systems. It ensures proper cleanup and deallocation of resources associated with the object.
The execution of destructors happens transparently behind the scenes, managed by the runtime environment or garbage collector, without requiring explicit invocation from the programmer. The destructor ensures that any necessary cleanup tasks are performed, contributing to the overall efficiency and stability of the program.
Declaration of Destructors
Destructors are optional. Destructors cannot return any values; nor can they accept any parameters. Unlike constructors, you cannot have more than one destructor defined for a class.
Conclusion
Destructors are a critical component in managing the lifecycle of objects and ensuring proper resource management. Their automatic execution simplifies memory and resource handling, reducing the risk of memory leaks and other resource-related issues.
- What are the different types of inheritance ?
- What are Access Modifiers ?
- Why Classes cannot be declared as Protected?
- Can we declare private class in namespace
- Difference between Classes and Structures
- Can we use pointers in C# ?
- Why abstract class can't create instance
- Can you prevent your class from being inherited
- Difference between method Overloading and Overriding
- Difference between Early Binding and Late binding
- What is nested class
- What is partial class ?
- What is Virtual Method
- Difference between class and object
- What is Data Encapsulation?
- Object Based Language and OOPs
- SOLID Principles in C#
- Solid Principles | Advantages and Disadvantages