Method Overloading and Method Overriding
Method overloading and method overriding are two important concepts in object-oriented programming, particularly in .NET. They both involve defining multiple methods with the same name but with different parameters or behaviors. However, there are significant differences between method overloading and method overriding. Let's examine each concept in detail with examples:
Method Overloading
Method overloading allows you to define multiple methods with the same name but with different parameters within the same class or in a derived class. The compiler differentiates between these methods based on their parameter types, number, or order.
Example | Method overloading: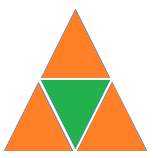
In the above example, the Calculator class has two Add methods: one that accepts two integers and another that accepts two doubles. The methods have the same name but different parameter types (integers vs. doubles). This allows the user to call the appropriate method based on the types of arguments they provide.
Method overloading provides flexibility and allows you to write code that can handle different types of data while using the same method name.
Method Overriding
Method overriding is a concept that occurs in inheritance, where a derived class provides a different implementation for a method that is already defined in its base class. The method in the derived class must have the same name, return type, and parameters as the method in the base class.
Example | Method overriding: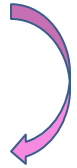
In the above example, the Shape class has a virtual method called Draw(). The Circle class inherits from Shape and overrides the Draw() method with its own implementation. The override keyword is used to indicate that the method is intended to override a base class method.
In this case, when the Draw() method is called on a Shape object that references a Circle instance, the overridden Draw() method in the Circle class is executed. This is known as runtime polymorphism or dynamic method dispatch.
Method overriding allows you to provide specialized behavior in derived classes while using the common interface defined in the base class.
Conclusion
Method overloading involves defining multiple methods with the same name but different parameters within the same class or in a derived class. Method overriding occurs in inheritance and allows a derived class to provide a different implementation for a method that is already defined in its base class.
- What is object-oriented programming?
- What is a Class?
- What is an Object?
- Constructors and Destructors
- What Is Inheritance ?
- What are the different types of inheritance ?
- What are Access Modifiers ?
- Why Classes cannot be declared as Protected?
- Can we declare private class in namespace
- Difference between Classes and Structures
- Can we use pointers in C# ?
- Why abstract class can't create instance
- Can you prevent your class from being inherited
- Difference between Early Binding and Late binding
- What is nested class
- What is partial class ?
- What is Virtual Method
- Difference between class and object
- What is Data Encapsulation?
- Object Based Language and OOPs
- SOLID Principles in C#
- Solid Principles | Advantages and Disadvantages