Can we use pointers in C# ?
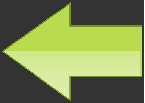
What is a pointer ?
A pointer is a variable that stores a memory address. Every variable declared in a program has two components:
- Address of a variable
- Value stored in the variable
Pointer declarations use the * operator.
E.g.
Is there pointer in C# like C or C++ ?
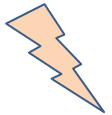
One of the biggest advantages of the .NET platform is the support for type safety. To maintain type safety and security, use of pointers is rarely required in C#. But, there are some situations that require them.
By using the unsafe keyword, you can define an unsafe context in which pointers can be used in .Net platform. Using an unsafe context to allow pointers is warranted by the following cases:
- Dealing with existing structures on disk.
- Advanced COM or Platform Invoke scenarios that involve structures with pointers in them.
- Performance-critical code.
In an unsafe context, a pointer type declaration takes one of the following forms:
Ex:
Note: Pointer types do not inherit from Object and there is no conversions exist between pointer types and Object.
Unsafe code
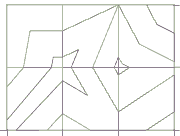
Unsafe code (unmanaged code ) allows you to manipulate memory directly. In the .NET environment, this is seen as potentially dangerous, and as such you have to mark your code as unsafe. Code written using an unsafe context cannot be verified to be safe, so it will be executed only when the code is fully trusted. That means, unsafe code cannot be executed in an untrusted environment, on the other hand, Managed Code has executed by the Common Language Runtime (CLR) environment. Click the following link to know more about .... Difference between managed and unmanaged code
- What is object-oriented programming?
- What is a Class?
- What is an Object?
- Constructors and Destructors
- What Is Inheritance ?
- What are the different types of inheritance ?
- What are Access Modifiers ?
- Why Classes cannot be declared as Protected?
- Can we declare private class in namespace
- Difference between Classes and Structures
- Why abstract class can't create instance
- Can you prevent your class from being inherited
- Difference between method Overloading and Overriding
- Difference between Early Binding and Late binding
- What is nested class
- What is partial class ?
- What is Virtual Method
- Difference between class and object
- What is Data Encapsulation?
- Object Based Language and OOPs
- SOLID Principles in C#
- Solid Principles | Advantages and Disadvantages