Difference between Classes and Structures
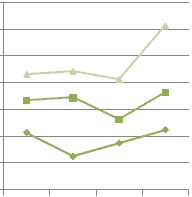
Classes and structures are both fundamental building blocks in object-oriented programming, but they have some key differences in terms of their behavior, usage, and memory allocation. Let's explore these differences in detail along with examples.
Definition and Usage
Classes:
A class is a reference type that serves as a blueprint for creating objects. It encapsulates data and behavior (methods) into a single entity. Classes are commonly used for modeling complex entities, implementing business logic, and creating reusable components in larger software systems.
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
public void SayHello()
{
Console.WriteLine("Hello, I'm " + Name);
}
}
Structures:
A structure is a value type that represents a lightweight container for storing related data. It is typically used for small data structures that don't require complex behavior or inheritance. Structures are commonly used for representing simple entities or for performance-critical scenarios where memory allocation and copying should be minimized.
public struct Point
{
public int X { get; set; }
public int Y { get; set; }
}
Memory Allocation and Performance
- Classes:Objects created from classes are stored on the heap, and a reference (memory address) to the object is stored on the stack or in another object. Memory allocation and deallocation for classes are managed by the garbage collector. When passing a class object as a method parameter or assigning it to another variable, the reference is passed, not the actual object. This can result in more memory overhead and potential performance impact.
- Structures:Structure instances are typically stored on the stack rather than the heap. They are allocated inline wherever they are declared or as part of another object. When you assign a structure to a new variable or pass it as a method parameter, a copy of the entire structure is made. This can lead to better performance for small, lightweight data structures, as it avoids the overhead of heap memory allocation and garbage collection.
Inheritance and Polymorphism
- Classes: Classes support inheritance, allowing one class to derive properties and behavior from another class. This enables code reuse and promotes the creation of hierarchical relationships. Classes can also participate in polymorphism, where objects of derived classes can be treated as objects of their base classes.
- Structures:Structures do not support inheritance or polymorphism. They cannot inherit from other structures or classes, and they cannot be used in place of their base types.
Default Initialization and Nullability
- Classes: Class objects are reference types and are initialized to a null reference by default if not explicitly initialized. This means that you can have a class object with no value assigned to it.
- Structures: Structure instances are value types and are automatically initialized with default values if not explicitly initialized. This means that a structure object always contains a valid value, even if it's a default value (e.g., 0 for numeric types).
Usage Guidelines
Use classes when you need to model complex entities, create reusable components, or take advantage of inheritance and polymorphism.
Use structures for lightweight data containers, simple entities, or performance-critical scenarios where memory allocation and copying should be minimized.
Conclusion
It's important to choose between classes and structures based on your specific needs and considerations related to memory usage, performance, behavior, and code design.
Related Topics
- What is object-oriented programming?
- What is a Class?
- What is an Object?
- Constructors and Destructors
- What Is Inheritance ?
- What are the different types of inheritance ?
- What are Access Modifiers ?
- Why Classes cannot be declared as Protected?
- Can we declare private class in namespace
- Can we use pointers in C# ?
- Why abstract class can't create instance
- Can you prevent your class from being inherited
- Difference between method Overloading and Overriding
- Difference between Early Binding and Late binding
- What is nested class
- What is partial class ?
- What is Virtual Method
- Difference between class and object
- What is Data Encapsulation?
- Object Based Language and OOPs
- SOLID Principles in C#
- Solid Principles | Advantages and Disadvantages