What is Virtual Method
In object-oriented programming, a virtual method is a method that can be overridden in derived classes. It allows subclasses to provide their own implementation of the method while still maintaining the same method signature as the base class. Virtual methods play a crucial role in achieving polymorphism and facilitating dynamic method dispatch. Here's a detailed explanation with examples:
Virtual Method
To define a virtual method in C#, you use the virtual keyword in the method declaration within the base class. The derived classes can then override this method with their own implementation.
In the above example, the Draw() method in the Shape class is declared as virtual. This allows derived classes, such as Circle, to override and provide their own implementation of the method using the override keyword.
Polymorphism and Dynamic Method Dispatch
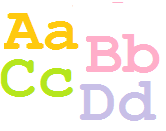
Virtual methods enable polymorphism, which means that a method call on a base class reference can be dynamically dispatched to the appropriate derived class method at runtime based on the actual object type.
In the above example, a Circle object is instantiated, but it is assigned to a Shape reference variable. When the Draw() method is called on the shape object, the overridden Draw() method in the Circle class is executed. This dynamic method dispatch ensures that the appropriate method implementation is invoked based on the actual object type.
Non-Virtual Methods
By default, methods in C# are non-virtual, meaning they cannot be overridden in derived classes unless explicitly marked as virtual. Non-virtual methods are resolved at compile-time, and the method called depends on the reference type rather than the actual object type.
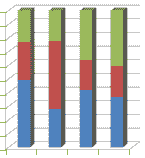
In the above example, the StartEngine() method in the Vehicle class is not marked as virtual. If you have a reference of type Vehicle pointing to a Car object and call StartEngine(), the method in the Vehicle class will be executed, not the one in the Car class.
To enable method overriding and dynamic dispatch, the base class method should be declared as virtual, and the derived class should use the override keyword to provide its own implementation.
Conclusion
Virtual methods are essential for achieving polymorphism and enabling flexible behavior in object-oriented programming. They allow derived classes to override base class methods and provide their own specialized implementation, promoting code extensibility and modularity.
- What is object-oriented programming?
- What is a Class?
- What is an Object?
- Constructors and Destructors
- What Is Inheritance ?
- What are the different types of inheritance ?
- What are Access Modifiers ?
- Why Classes cannot be declared as Protected?
- Can we declare private class in namespace
- Difference between Classes and Structures
- Can we use pointers in C# ?
- Why abstract class can't create instance
- Can you prevent your class from being inherited
- Difference between method Overloading and Overriding
- Difference between Early Binding and Late binding
- What is nested class
- What is partial class ?
- Difference between class and object
- What is Data Encapsulation?
- Object Based Language and OOPs
- SOLID Principles in C#
- Solid Principles | Advantages and Disadvantages