How to Get the Current Date in JavaScript
The Date object in JavaScript represents a date and time, internally represented as the number of milliseconds elapsed since the epoch - midnight on January 1, 1970, UTC. JavaScript usually utilizes the time zone of the browser, displaying dates in the local time zone by default. When displayed, the date is typically shown in a full text string format, making it human-readable.
Date objects in JavaScript
JavaScript's Date objects are generated using the new Date() constructor. This function instantiates a new date object that corresponds to the current date and time. The Date object offers a variety of methods to manipulate and extract information from dates. The particular method you utilize will depend on the desired format of the date information you intend to obtain. This flexibility allows you to work with dates in diverse ways, accommodating different use cases and formatting requirements.
Simple JavaScript Date operation

If you want to get Day, Month and Year separate then you can use the following code.

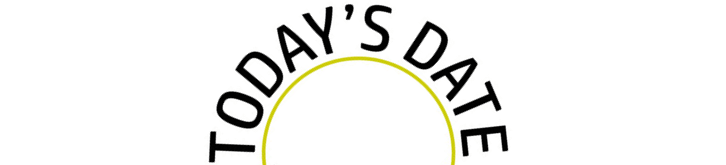
Using Date.prototype.toLocaleDateString()
The toLocaleDateString() method is a built-in function in JavaScript's Date object that returns a string presenting the date part of the specified date, utilizing the local language-sensitive formatting rules. This method also takes into account the user agent's time zone, ensuring the representation of the date is appropriate for the user's region.

Using Date.prototype.toISOString()
The toISOString() method in JavaScript's Date object returns the date as a string, adhering to the ISO-8601 standard format. This format is represented as "YYYY-MM-DDTHH:mm:ss.sssZ," where:
- YYYY represents the year.
- MM represents the month.
- DD represents the day.
- THH represents the hour.
- mm represents the minute.
- ss represents the second.
- sss represents the milliseconds.
- Z represents the UTC time zone.
The toISOString() method produces a standardized string representation that's commonly used for data interchange and storage across different systems.

Detailed date format
You can try this detailed date format like day name , month name etc.

Finally, you can use a single line code using JSON for getting the current date.

Moreover, you can use Date.js library which extends Date object , thus you can have .today() method.
Conclusion
To acquire the current date in JavaScript, create a Date object without parameters, and then use the object's methods to extract the required components. This enables you to work with and display the current date and time effectively.
- JavaScript Popup Boxes
- Opening a new window in JavaScript
- How to Create Drop-Down Lists in JavaScript
- How do I include a JavaScript file in another JavaScript file?
- Print the content of a Div using JavaScript/jQuery
- How to get the current URL using JavaScript ?
- How to Detect a Mobile Device with JavaScript/jQuery
- How to validate an email address in JavaScript
- JavaScript Array Iteration
- How to Remove a Specific Item from an Array in JavaScript
- What is JavaScript closures?
- How To Remove a Property from a JavaScript Object
- How to get selected value from Dropdown list in JavaScript
- How to Open URL in New Tab | JavaScript
- How to delay/wait/Sleep in code execution | JavaScript
- How to round to at most 2 decimal places | JavaScript
- How to convert string to boolean | JavaScript
- How to check undefined in JavaScript?
- How To Copy to Clipboard | JavaScript
- How to encode a URL using JavaScript?
- How to force Input field to enter numbers only | JavaScript
- How to create multiline string in JavaScript
- How to Check for an Empty String in JavaScript?