What is the best way to detect a mobile device
Checking for a mobile device in a web app is a common requirement, but relying solely on user agent detection is not the best approach for modern web applications. Thankfully, there's a more robust alternative using the built-in JavaScript API for media detection.
window.matchMedia() method
The window.matchMedia() method serves as a valuable tool, allowing developers to create responsive designs by evaluating CSS media queries directly. This method returns a MediaQueryList object that reflects the outcome of a specified CSS media query string. By employing this approach, you can accurately detect mobile devices and their characteristics, enhancing the adaptability and user experience of your web application.
You can use above script to do show/hide elements depending on the screen size.
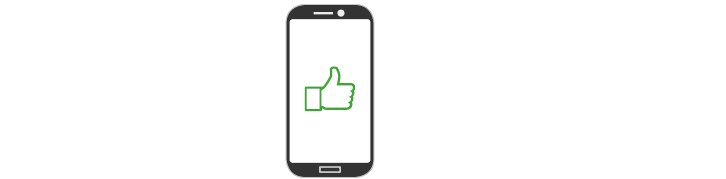
Use Agent Detection (Not Recommended)
While using the user agent to detect mobile devices is relatively straightforward, it's not the most reliable method due to its susceptibility to manipulation. User agent strings can indeed be spoofed easily, which can lead to inaccurate results. Despite its simplicity, this method lacks the robustness needed for modern web development, particularly when security and accurate device detection are essential.
To get the user agent string, you can use the navigator.userAgent property.
The userAgent value can be altered quite easily, even intentionally, which makes it an unreliable method for accurately identifying the actual device type. Bots and scrapers, among other tools, can manipulate the user agent value to conceal their true identity or characteristics. As a result, relying solely on the userAgent for device detection becomes even less trustworthy, emphasizing the need for more robust and secure techniques in modern web development.
Conclusion
To detect a mobile device using JavaScript, the user agent string is analyzed with a regular expression. This technique enables you to differentiate between mobile and non-mobile devices and tailor your website or application accordingly.
- JavaScript Popup Boxes
- Opening a new window in JavaScript
- How to Create Drop-Down Lists in JavaScript
- How do I include a JavaScript file in another JavaScript file?
- Print the content of a Div using JavaScript/jQuery
- How to get the current URL using JavaScript ?
- How to validate an email address in JavaScript
- JavaScript Array Iteration
- How to Remove a Specific Item from an Array in JavaScript
- What is JavaScript closures?
- How To Remove a Property from a JavaScript Object
- How to get selected value from Dropdown list in JavaScript
- How do I get the current date in JavaScript?
- How to Open URL in New Tab | JavaScript
- How to delay/wait/Sleep in code execution | JavaScript
- How to round to at most 2 decimal places | JavaScript
- How to convert string to boolean | JavaScript
- How to check undefined in JavaScript?
- How To Copy to Clipboard | JavaScript
- How to encode a URL using JavaScript?
- How to force Input field to enter numbers only | JavaScript
- How to create multiline string in JavaScript
- How to Check for an Empty String in JavaScript?