Print the content of a Div | JavaScript/jQuery
Printing an entire web page is a built-in feature provided by web browsers. Yet, there are scenarios where you might require users to print a specific segment, such as the content within a <div> , rather than the entire page. This targeted printing can be achieved through JavaScript, enabling more fine-grained control over what gets printed.
In the above code, demonstrates a process where clicking the "Print a Div" button initiates the creation of a JavaScript popup window. This window is utilized to extract and store the contents of HTML <div> elements within a JavaScript variable. Subsequently, the content of these HTML elements is inscribed into the popup window, and the final step involves printing the window using the JavaScript window.print() command. This sequence of actions facilitates the selective printing of specific content from the webpage.

Print This Image...
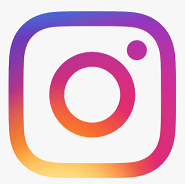
Full Source | JavaScript
Conclusion
Printing the content of a <div> using JavaScript involves extracting the content with innerHTML and creating a new window to display it. This approach enables users to initiate printing through the browser's print functionality.
- JavaScript Popup Boxes
- Opening a new window in JavaScript
- How to Create Drop-Down Lists in JavaScript
- How do I include a JavaScript file in another JavaScript file?
- How to get the current URL using JavaScript ?
- How to Detect a Mobile Device with JavaScript/jQuery
- How to validate an email address in JavaScript
- JavaScript Array Iteration
- How to Remove a Specific Item from an Array in JavaScript
- What is JavaScript closures?
- How To Remove a Property from a JavaScript Object
- How to get selected value from Dropdown list in JavaScript
- How do I get the current date in JavaScript?
- How to Open URL in New Tab | JavaScript
- How to delay/wait/Sleep in code execution | JavaScript
- How to round to at most 2 decimal places | JavaScript
- How to convert string to boolean | JavaScript
- How to check undefined in JavaScript?
- How To Copy to Clipboard | JavaScript
- How to encode a URL using JavaScript?
- How to force Input field to enter numbers only | JavaScript
- How to create multiline string in JavaScript
- How to Check for an Empty String in JavaScript?