Multiple Inheritance in C#
No, C# does not support multiple inheritance for classes. Multiple inheritance refers to the ability of a class to inherit from more than one base class. In C#, a class can only inherit from a single base class.
Interfaces
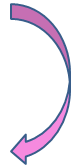
However, C# does support multiple inheritance through interfaces. An interface defines a contract that a class can implement, and a class can implement multiple interfaces. This allows a class to inherit behavior from multiple sources.
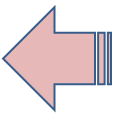
In the above example, the class MyClass implements both IInterface1 and IInterface2, allowing it to inherit and provide implementations for the methods defined in both interfaces.
Conclusion
Although C# does not support multiple inheritance for classes, it provides the concept of interfaces to achieve similar functionality and flexibility by allowing a class to implement multiple interfaces and inherit behavior from multiple sources.
- What is Process ID ?
- How do I make a DLL in C# ?
- How many ways you can pass values to Windows Services ?
- Can we use "continue" statement in finally block ?
- What is nullable type in c# ?
- Difference between the Debug class and Trace class ?
- What is lock statement in C#
- What are dynamic type variables in C#
- What is the difference between is and as operator in C#?
- What are circular references in C#?
- What are the differences between events and delegates in C#
- Explain the types of unit test cases in C#?
- How many types of comments are there in C#?
- What are the various ways to pass parameters to a method in C#?
- What are the different ways to deploy a assembly in net?
- What does assert() method do in c#
- What is literals in C# - Constants and Literals
- What is the use of goto statement in C#
- How can JIT code be faster than AOT compiled code
- Why events have no return types in .NET
- What's the difference between a static method and a non-static method in C#
- What's a weak reference c#?
- What is C# equivalent of the vb.net isNothing function
- What are indexers in C#
- What are generics in c#