Literals and Constants in C#
Literal is a source code representation of a value, i.e. it is a value that has been hard-coded directly into your source.
Examplex is a variable, and 100 is a literal.
Type of literals in C# are:
- Boolean Literal
- Integer Literal
- Real Literal
- Character Literal
- String Literal
- Null Literal
Boolean Literal
There are two Boolean literal values: true and false.
ExampleInteger Literal
Integer literals are used to write values of types int, uint, long, and ulong. Integer literals have two possible forms: decimal and hexadecimal.
ExampleReal Literal
Real literals are used to write values of types float, double, and decimal.
ExampleCharacter Literal
A character literal represents a single character, and usually consists of a character in quotes, as in 'a'.
Example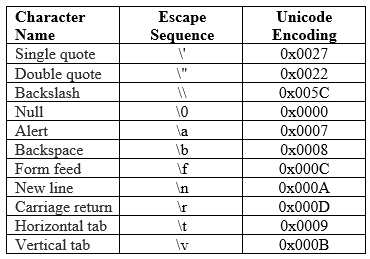
String Literal
C# provides support for two types of string literals: regular string literals and verbatim string literals.
Regular string literals are enclosed within double quotes, such as "string". They can contain any sequence of characters, including escape sequences like "\t" for the tab character or "\n" for a new line. Additionally, regular string literals can also include hexadecimal or Unicode escape sequences to represent specific characters.
On the other hand, verbatim string literals are denoted by an "@" character preceding the opening double-quote, such as @"string". They allow for the inclusion of escape sequences and characters without the need for special handling. This means that backslashes "" within verbatim string literals are treated as ordinary characters, and no additional escaping is required. Verbatim string literals are particularly useful when working with file paths, regular expressions, or any scenario where backslashes or escape sequences are common.
ExampleNull Literal
Null Literal is literal that denotes null type. Moreover, null can fit to any reference-type . and hence is a very good example for polymorphism.
ExampleConstants
Constants are values which are fixed and cannot be changed anytime during program execution. They can be of any data type.
- Does C# support multiple Inheritance ?
- What is Process ID ?
- How do I make a DLL in C# ?
- How many ways you can pass values to Windows Services ?
- Can we use "continue" statement in finally block ?
- What is nullable type in c# ?
- Difference between the Debug class and Trace class ?
- What is lock statement in C#
- What are dynamic type variables in C#
- What is the difference between is and as operator in C#?
- What are circular references in C#?
- What are the differences between events and delegates in C#
- Explain the types of unit test cases in C#?
- How many types of comments are there in C#?
- What are the various ways to pass parameters to a method in C#?
- What are the different ways to deploy a assembly in net?
- What does assert() method do in c#
- What is the use of goto statement in C#
- How can JIT code be faster than AOT compiled code
- Why events have no return types in .NET
- What's the difference between a static method and a non-static method in C#
- What's a weak reference c#?
- What is C# equivalent of the vb.net isNothing function
- What are indexers in C#
- What are generics in c#