How do I make a DLL in C#
To create a DLL (Dynamic Link Library) in C#, you can follow these steps:
Step 1: Create a new Class Library project in Visual Studio
- Open Visual Studio and go to "File" > "New" > "Project".
- Select "Class Library (.NET Framework)" or "Class Library (.NET Core)" project template, depending on your target framework.
- Choose a suitable project name and location, and click "OK" to create the project.
Step 2: Add classes and functionality to the DLL
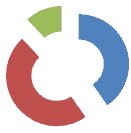
- Add the necessary classes, interfaces, and other types to the project.
- Implement the desired functionality within these classes.
- Consider the intended purpose of your DLL and design the classes accordingly.
In the above example, we define a Calculator class with an Add method that performs addition. We also define an ILogger interface.
Step 3: Build the project to generate the DLL
- Build the project by selecting "Build" > "Build Solution" or pressing Ctrl + Shift + B.
- The build process will generate the DLL file, typically with the extension .dll, in the output folder of the project.
Step 4: Use the DLL in other projects or applications
- To use the DLL in another project or application, you need to add a reference to the DLL.
- In your target project, right-click on "References" and select "Add Reference".
- Browse to the location of the DLL and select it to add the reference.
Now, you can use the classes and functionality provided by the DLL in your project.
Example usage:In the above example, we reference the MyLibrary DLL (assuming that is the name of our DLL project) and use the Calculator class to perform addition.
Conclusion
Creating a DLL in C# involves creating a Class Library project, implementing the desired functionality within classes, and building the project to generate the DLL file. The resulting DLL can then be referenced and used in other projects or applications, providing the encapsulated functionality and types to the consuming code.
- Does C# support multiple Inheritance ?
- What is Process ID ?
- How many ways you can pass values to Windows Services ?
- Can we use "continue" statement in finally block ?
- What is nullable type in c# ?
- Difference between the Debug class and Trace class ?
- What is lock statement in C#
- What are dynamic type variables in C#
- What is the difference between is and as operator in C#?
- What are circular references in C#?
- What are the differences between events and delegates in C#
- Explain the types of unit test cases in C#?
- How many types of comments are there in C#?
- What are the various ways to pass parameters to a method in C#?
- What are the different ways to deploy a assembly in net?
- What does assert() method do in c#
- What is literals in C# - Constants and Literals
- What is the use of goto statement in C#
- How can JIT code be faster than AOT compiled code
- Why events have no return types in .NET
- What's the difference between a static method and a non-static method in C#
- What's a weak reference c#?
- What is C# equivalent of the vb.net isNothing function
- What are indexers in C#
- What are generics in c#