Generic in C# with Examples
Generic means the general form, not specific. In C#, generic means not specific to a particular data type, as defined by. C# allows developers to define generic classes, interfaces, abstract classes, fields, methods, static methods, properties, events, delegates, and operators using the type parameter and without the specific data type, as stated in.
In other words, with generics, you can define a class or method that can work with different types of data without having to write separate code for each type. Instead, you can create a single generic class or method that can be used with any data type, making your code more flexible and reusable.
Generic Class
In C#, a generic class is a class that is parameterized by one or more type parameters, which are used to define the types of fields, properties, methods, and other members of the class. Generic classes provide a way to write code that can work with different types without having to rewrite the same code for each type.
The syntax for declaring a generic class in C# is similar to that of a regular class, except that the class name is followed by one or more type parameters enclosed in angle brackets "<>".
A simple generic class in C#
In the above code, the type parameter "T" is used to define the type of the private field "_myField" and the public property "MyProperty". The constructor of the class takes a parameter of type "T", which is used to initialize the "_myField" field. The class can be instantiated with any type, and the type of the field and property will be determined at runtime based on the type argument provided when the class is instantiated.
Generic Methods
C# generic methods are methods that can be parameterized by one or more type parameters. These type parameters can be used to define the types of the method's parameters, return values, or local variables within the method's body.
By using generic methods, you can create methods that can work with different types of data without having to write separate methods for each type. For example, a generic method to sort an array of integers could also be used to sort an array of strings or any other type that implements the IComparable interface.
Following is an example of a simple generic method that takes two arguments of the same type and returns the larger of the two:
In the above code, the method is parameterized by a type parameter T, which is constrained to implement the IComparable interface. This constraint ensures that the CompareTo method can be called on the type parameter T to compare the two arguments.
You can call this method with any type that implements the IComparable interface, such as int, double, or string, and the method will return the larger of the two arguments.
Generic Field
In C#, a generic field is a field that is defined with a type parameter, allowing it to store any type of value that satisfies the constraints defined by the type parameter.
In this code, the class MyClass has a generic field myField of type T. The type T is defined as a type parameter when the class is defined, which means that any value of type T can be stored in myField. When an instance of MyClass is created, a value is passed to the constructor, which sets the value of myField to that value. The GetFieldValue method allows the value of myField to be retrieved.
Using a generic field can make a class more flexible and reusable, as it allows it to work with a wider range of types.
C# Generic | Example
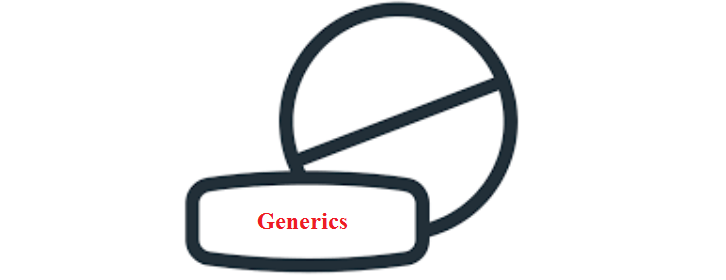
Following is an example program that uses a generic class, method, and field:
In the above program, define a generic class called MyGenericClass that has a generic field, myField, which can store any type of data. The class also has a generic method, Add, that can perform addition on the data stored in myField, and another generic method, Concatenate, that can concatenate two strings.
In the Main method, create an instance of MyGenericClass with int as the type parameter and another instance with string as the type parameter. Then call the Add and Concatenate methods on these instances, passing in appropriate values, and print the results to the console.
Advantages of C# Generics
There are several advantages of using C# generics, including:
- Type Safety: Generics provide type safety by allowing you to specify the type of data a collection or class can hold. This helps to catch errors at compile-time, rather than at run-time, which makes your code more reliable and easier to debug.
- Code Reusability: Generics allow you to write reusable code that can work with different types of data. By writing a generic class or method, you can avoid duplicating code for different types, and you can use the same code to work with a variety of data types.
- Performance: Generics provide better performance compared to non-generic code. This is because the runtime does not need to box and unbox values, which can be expensive in terms of performance.
- Scalability: Generics can help to improve the scalability of your code. With generics, you can write code that can handle large datasets without having to rewrite the code for each type of data. This makes it easier to scale your code as your data grows.
- Code Clarity: Generics can make your code more readable and easier to understand. By specifying the type of data that a collection or class can hold, you can make your code more self-documenting, which makes it easier for other developers to understand your code.
Conclusion:
Generic classes are very powerful and flexible, and they are widely used in C# to write reusable code that can work with different types.
- Does C# support multiple Inheritance ?
- What is Process ID ?
- How do I make a DLL in C# ?
- How many ways you can pass values to Windows Services ?
- Can we use "continue" statement in finally block ?
- What is nullable type in c# ?
- Difference between the Debug class and Trace class ?
- What is lock statement in C#
- What are dynamic type variables in C#
- What is the difference between is and as operator in C#?
- What are circular references in C#?
- What are the differences between events and delegates in C#
- Explain the types of unit test cases in C#?
- How many types of comments are there in C#?
- What are the various ways to pass parameters to a method in C#?
- What are the different ways to deploy a assembly in net?
- What does assert() method do in c#
- What is literals in C# - Constants and Literals
- What is the use of goto statement in C#
- How can JIT code be faster than AOT compiled code
- Why events have no return types in .NET
- What's the difference between a static method and a non-static method in C#
- What's a weak reference c#?
- What is C# equivalent of the vb.net isNothing function
- What are indexers in C#