Lock and Monitor in C#
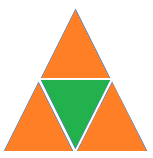
The Lock statement is commonly used in multithreading scenarios to restrict the concurrent execution of a specific activity or section of code by multiple threads. It ensures exclusive access to a critical section, preventing multiple threads from entering it simultaneously.
When using a lock statement, a thread acquires a monitor by taking a reference to an object associated with the critical section. This object acts as a synchronization mechanism, often referred to as a "monitor". Only one thread can acquire a specific monitor at any given time. If a second thread attempts to acquire the same monitor while another thread is holding it, it will be blocked and wait until the monitor becomes available. This mechanism ensures that conflicting access to shared resources is avoided and maintains data integrity.
Upon completing the execution of the critical section, the thread releases the lock, allowing other threads to acquire the monitor and proceed with their own execution. Releasing the lock ensures that other threads can access the critical section in a serialized and synchronized manner, promoting thread safety.
Example: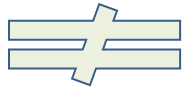
When utilizing the lock statement in C#, only one thread can acquire the lock on an object at a given time. If multiple threads attempt to acquire the lock simultaneously, they are placed in a queue known as the "ready queue" and are granted the lock on a first-come, first-served basis. This queuing mechanism ensures that threads waiting to acquire the lock are given access in the order they requested it.
In the provided code snippet, the first thread enters the critical section and acquires the lock on myObj. When another thread attempts to enter the critical section, it also tries to acquire the lock on myObj. However, since the lock is already held by the first thread, the second thread is blocked and must wait for the first thread to release the lock.
Once the first thread completes its execution in the critical section and releases the lock on myObj, the second thread can then acquire the lock and enter the critical section to perform its own operations.
This queuing behavior ensures that threads have synchronized access to the critical section and prevents concurrent modification of shared resources, maintaining data integrity and preventing race conditions.
Monitor.Enter and Monitor.Exit
The lock statement is in fact a syntactic shortcut for a call to the methods Monitor.Enter and Monitor.Exit, with a try/finally block.
The lock keyword calls Enter at the start of the block and Exit at the end of the block and it actually handles Monitor class at back end. It is legal for the same thread to invoke Enter more than once without it blocking; however, an equal number of Exit calls must be invoked before other threads waiting on the object will unblock. Calling Monitor.Exit without first calling Monitor.Enter on the same object throws an exception.
Nested Locking
A thread can repeatedly lock the same object in a nested fashion.
In the above case, the object is unlocked only when the outermost lock statement has exited.
Deadlocks
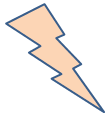
A deadlock is a situation that occurs in multithreading when two or more threads are blocked indefinitely, waiting for each other to release resources that they hold. It creates a situation where none of the threads can proceed with their execution.
Deadlocks typically happen when multiple threads contend for shared resources, and each thread holds a resource that is required by another thread. This results in a circular dependency where neither thread can release the resource it holds because it is waiting for the other thread to release the resource it needs.
Here's an example to illustrate a deadlock scenario:
Thread 1:Acquires lock on Resource A.
Needs access to Resource B.
Thread 2:Acquires lock on Resource B.
Needs access to Resource A.
In this case, both threads are waiting for a resource held by the other thread. As a result, a deadlock occurs, and neither thread can proceed further.
Deadlocks can arise due to various factors, such as improper resource locking, incorrect synchronization, or race conditions. They are particularly challenging to debug and resolve because they often involve complex interactions between multiple threads.
To prevent deadlocks, it is essential to carefully manage resource locking and synchronization. This involves analyzing the dependencies between threads and resources, ensuring proper ordering of lock acquisition, and using synchronization constructs like timeouts or avoiding circular dependencies altogether.
Detecting and resolving deadlocks often requires techniques such as deadlock detection algorithms, resource allocation graphs, or designing thread-safe algorithms that minimize resource contention.
Check ths simple example to understand DeadLock very clearly:
In the above program :
- FirstThread acquires firstLock.
- SecondThread acquires secondLock.
- FirstThread attempts to acquire secondLock, but it is already held by SecondThread and thus FirstThread blocks until
- SecondLock is released.
- SecondThread attempts to acquire firstLock, but it is held by FirstThread and thus SecondThread blocks until firstLock is released.
At this point, both threads are blocked and will never wake up.
What is lock statement in VB.Net?
Is there a lock statement in VB.NET ?Yes, it is called SyncLock
SyncLock prevents each thread from entering the block until no other thread is executing it.
VB.Net Example:
Conclusion
The lock statement in C# ensures that only one thread can hold the lock on an object at any given time. Contending threads are queued and granted access on a first-come, first-served basis, ensuring orderly and synchronized access to critical sections. This mechanism helps prevent data corruption and race conditions when multiple threads access shared resources.
- Does C# support multiple Inheritance ?
- What is Process ID ?
- How do I make a DLL in C# ?
- How many ways you can pass values to Windows Services ?
- Can we use "continue" statement in finally block ?
- What is nullable type in c# ?
- Difference between the Debug class and Trace class ?
- What are dynamic type variables in C#
- What is the difference between is and as operator in C#?
- What are circular references in C#?
- What are the differences between events and delegates in C#
- Explain the types of unit test cases in C#?
- How many types of comments are there in C#?
- What are the various ways to pass parameters to a method in C#?
- What are the different ways to deploy a assembly in net?
- What does assert() method do in c#
- What is literals in C# - Constants and Literals
- What is the use of goto statement in C#
- How can JIT code be faster than AOT compiled code
- Why events have no return types in .NET
- What's the difference between a static method and a non-static method in C#
- What's a weak reference c#?
- What is C# equivalent of the vb.net isNothing function
- What are indexers in C#
- What are generics in c#