Can we use "continue" statement in finally block
No, it is not possible to use the continue statement within a finally block in C#. The finally block is used for cleanup operations and is executed after the try block or any associated catch blocks, regardless of whether an exception was thrown or not.
Continue statement
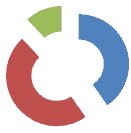
The continue statement is used within loop constructs (such as for, while, or do-while) to skip the current iteration and move to the next iteration of the loop. However, the finally block is not part of the loop construct, and its purpose is solely for cleanup operations, not controlling the flow of the loop.
Example illustrating the usage of finally and continue statements:
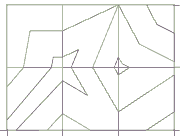
In the above example, the finally block is used for performing cleanup operations. However, attempting to use the continue statement within the finally block would result in a compilation error.
If you need to control the flow within a loop, including skipping iterations, you should use the continue statement within the loop construct itself (e.g., within the try block or after the catch block), but not inside the finally block.
Conclusion
The continue statement cannot be used within a finally block in C#. The finally block is intended for cleanup operations, and its execution is separate from loop control flow. If you need to skip iterations in a loop, use the continue statement within the loop construct itself, outside of the finally block.
- Does C# support multiple Inheritance ?
- What is Process ID ?
- How do I make a DLL in C# ?
- How many ways you can pass values to Windows Services ?
- What is nullable type in c# ?
- Difference between the Debug class and Trace class ?
- What is lock statement in C#
- What are dynamic type variables in C#
- What is the difference between is and as operator in C#?
- What are circular references in C#?
- What are the differences between events and delegates in C#
- Explain the types of unit test cases in C#?
- How many types of comments are there in C#?
- What are the various ways to pass parameters to a method in C#?
- What are the different ways to deploy a assembly in net?
- What does assert() method do in c#
- What is literals in C# - Constants and Literals
- What is the use of goto statement in C#
- How can JIT code be faster than AOT compiled code
- Why events have no return types in .NET
- What's the difference between a static method and a non-static method in C#
- What's a weak reference c#?
- What is C# equivalent of the vb.net isNothing function
- What are indexers in C#
- What are generics in c#