What is nullable type in c# ?
In C#, a nullable type allows you to assign an additional value, null, to value types such as integers, floats, booleans, etc., which by default cannot hold a null value. It provides a way to represent the absence of a value for value types.
Key points to about nullable types:
- Value Types vs. Reference Types: In C#, value types represent data directly, while reference types hold a reference to an object. Value types, by default, cannot be assigned a null value. However, by using nullable types, you can assign null to value types.
- Nullable Value Types: A nullable value type is a value type that can also have a null value. It is created by appending a ? to the type declaration. For example, int? represents a nullable integer, float? represents a nullable float, and so on.
- Nullable Value Type Properties: You can declare nullable value types as properties in classes or use them as method parameters. This allows for scenarios where a value might be missing or unknown.
- Nullable Value Type Operations: Nullable types allow you to perform operations on nullable value types using the null coalescing operator (??) or by checking for null using the HasValue property. This helps in handling scenarios where the value may or may not be null.
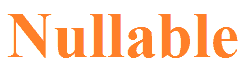
In the above example, nullableInt is a nullable integer that can hold either an integer value or null. We assign values to nullableInt and demonstrate checking for null using the HasValue property. We also use the null coalescing operator (??) to assign a default value of 0 to result if nullableInt is null.
Conclusion
The nullable types in C# allow you to assign null to value types, representing the absence of a value. They enable handling scenarios where a value may or may not be present. By using nullable types, you can work with value types that can hold null, perform null-checking operations, and provide more flexibility in your code.
- Does C# support multiple Inheritance ?
- What is Process ID ?
- How do I make a DLL in C# ?
- How many ways you can pass values to Windows Services ?
- Can we use "continue" statement in finally block ?
- Difference between the Debug class and Trace class ?
- What is lock statement in C#
- What are dynamic type variables in C#
- What is the difference between is and as operator in C#?
- What are circular references in C#?
- What are the differences between events and delegates in C#
- Explain the types of unit test cases in C#?
- How many types of comments are there in C#?
- What are the various ways to pass parameters to a method in C#?
- What are the different ways to deploy a assembly in net?
- What does assert() method do in c#
- What is literals in C# - Constants and Literals
- What is the use of goto statement in C#
- How can JIT code be faster than AOT compiled code
- Why events have no return types in .NET
- What's the difference between a static method and a non-static method in C#
- What's a weak reference c#?
- What is C# equivalent of the vb.net isNothing function
- What are indexers in C#
- What are generics in c#