Add new item to List object C#
The C# List is a dynamic data structure that allows you to add, remove, and access elements based on their index.
Following are some ways to add items to a C# List:
- Add method
- AddRange method
- Insert method
- InsertRange method
- List initializer syntax
Using the Add method
The Add method is the simplest way to add items to a C# List. It adds an item to the end of the list.
Using the AddRange method
The AddRange method allows you to add a collection of items to a C# List. It takes an IEnumerable
Using the Insert method
The Insert method allows you to add an item at a specific index in a C# List. It takes two arguments: the index where the item should be inserted and the item itself.
Using the InsertRange method
The InsertRange method allows you to add a collection of items at a specific index in a C# List. It takes two arguments: the index where the items should be inserted and the collection of items itself.
Using the List initializer syntax
The List initializer syntax allows you to add items to a C# List when it is initialized.
These are all the possible methods to add items to a C# List. Choose the one that best suits your needs based on the context of your code.
Possible Exceptions | C# list.add
In C#, when adding items to a List, there are several exceptions that may occur depending on the method used and the specific scenario.
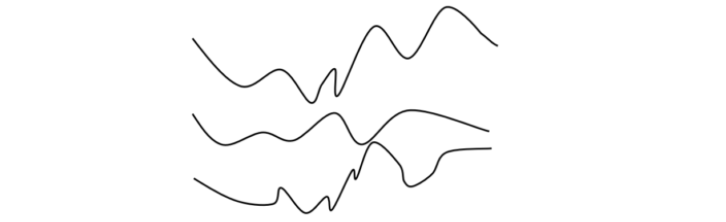
Following are some of the most common exceptions that can occur when adding items to a List in C#:
ArgumentNullException:
This exception occurs when you try to add a null item to the List.
To avoid this exception, you can check if the item is null before adding it to the List:
ArgumentOutOfRangeException:
This exception occurs when you try to insert an item at an index that is out of range.
To avoid this exception, you can check if the index is within the range of the List before inserting the item:
NotSupportedException:
This exception occurs when you try to add or insert an item to a List that is read-only or has a fixed size.
To avoid this exception, you can create a new List that is not read-only or has a variable size, and then add or insert items to it:
ArgumentException:
This exception occurs when you try to add an item to a List that has a duplicate value and the List does not allow duplicates.
To avoid this exception, you can use a HashSet instead of a List if you need to store unique values.
- Advantages of C#
- C# vs. Java: What Are Its Main Differences
- Advantages of C# over Python
- First C# Program | Hello World
- Difference between Console.Write and Console.WriteLine in C#
- How do I create a MessageBox in C#?
- C# Comments
- How to reverse a string in C#
- Palindrome in C# with Examples
- Fibonacci Series Program in C# with Examples
- C# Program to Print Number Triangle
- Get Integer Input from User in C# Console Application
- C# StringBuilder | Learn To Use C# StringBuilder Class
- C# HashMap (Dictionary)
- Ternary Operator (? :) in C# with Examples
- How To Sort Datatable in C#
- Struct Vs Class in C# | Differencees and Advantages
- Async And Await In C#
- IEnumerable in C# | Examples
- ref Vs out in C#
- How to remove item from list in C#?
- C# StreamWriter Examples
- StreamReader in C# |Examples
- C# Map Example
- Static Method In C# | Examples
- How to convert an Enum to a String in C#
- How to convert a string to an enum in C#
- How to filter a list in C#?