Triangle Patterns in C#
A number triangle is a type of pattern that displays a series of numbers in a triangular format. In C#, there are various methods to create a number triangle. Here are some possible methods:
Using nested loops
One way to create a number triangle in C# is by using nested loops.
Explanation:
First declare two variables n and num. n represents the number of rows and want to display, while num is the starting number for the triangle. Then use a for loop to iterate through each row of the triangle. The outer loop runs n times, one for each row. Within the outer loop, use another for loop to iterate through each column of the triangle. The inner loop runs i times, where i represents the current row number. Inside the inner loop, print the current value of num, followed by a space. Then increment num by 1. After the inner loop finishes, print a newline character to move to the next row.
Using recursion
Following is an example of how to generate a number triangle using recursion in C#:
Above code defines a TriangleNumber method that takes two arguments: the row number and the column number of a given position in the triangle. The method uses recursion to calculate the value at that position based on the values of the two positions above it. The main method then loops through each row and column of the triangle, calling the TriangleNumber method to generate the value at each position and printing it out. This produces the output of the number triangle:
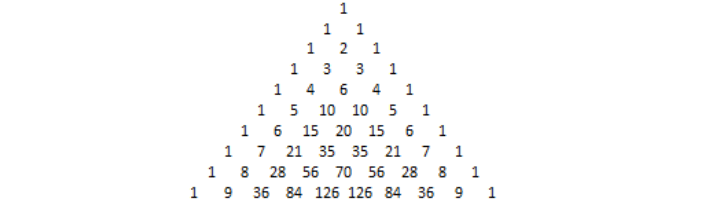
Note that the TriangleNumber method uses two base cases: when the column is 0 or when the column is equal to the row number. In both cases, the value at that position 1.
Using a single loop and arithmetic progression
Another method to create a number triangle is by using a single loop and arithmetic progression.
Explanation:
This method is similar to the nested loop method, but instead of using two loops, use a single loop to iterate through each row. Calculate the sum of the current row using the formula rowSum = (i * (i + 1)) / 2, where i is the current row number. Within the loop, print the current value of num, followed by a space. Then increment num by 1. After the loop finishes, print the sum of the current row.
- Advantages of C#
- C# vs. Java: What Are Its Main Differences
- Advantages of C# over Python
- First C# Program | Hello World
- Difference between Console.Write and Console.WriteLine in C#
- How do I create a MessageBox in C#?
- C# Comments
- How to reverse a string in C#
- Palindrome in C# with Examples
- Fibonacci Series Program in C# with Examples
- Get Integer Input from User in C# Console Application
- C# StringBuilder | Learn To Use C# StringBuilder Class
- C# HashMap (Dictionary)
- Ternary Operator (? :) in C# with Examples
- How To Sort Datatable in C#
- Struct Vs Class in C# | Differencees and Advantages
- Async And Await In C#
- IEnumerable in C# | Examples
- ref Vs out in C#
- How to remove item from list in C#?
- How to Add Items to a C# List
- C# StreamWriter Examples
- StreamReader in C# |Examples
- C# Map Example
- Static Method In C# | Examples
- How to convert an Enum to a String in C#
- How to convert a string to an enum in C#
- How to filter a list in C#?