How to read file using StreamReader in C#
StreamReader is a class in the .NET Framework that provides methods for reading character data from a stream. It is typically used to read text files, network streams, or any other stream that contains character data.
The StreamReader class provides various methods for reading characters from a stream, such as Read(), ReadLine(), and ReadToEnd(). These methods can be used to read data from the stream in various ways, depending on the needs of the application.
StreamReader is commonly used in conjunction with other classes in the .NET Framework, such as FileStream, NetworkStream, or MemoryStream. It can be used to read data from these streams and convert it into strings or other data types.
When using StreamReader, it's important to properly dispose of the object after it's no longer needed to avoid memory leaks. This can be done by calling the Dispose() method or by using the using statement to automatically dispose of the object when it goes out of scope.
StreamReader.Read() method
The StreamReader.Read() method is a method of the StreamReader class in C#, and it reads a single character from the current stream and advances the character position by one character. The method returns an integer value that represents the Unicode code point of the character read. If the end of the stream is reached, the method returns -1.
Following is an example of how you can use the StreamReader.Read() method to read a single character from a file:
In the above example, first create a StreamReader object that reads from the file "file.txt". Then, use a while loop to read each character from the file using the Read() method. Inside the loop, do something with the character (in this case, simply write it to the console), and then read the next character.
Note that the StreamReader.Read() method reads a single character at a time, so it can be less efficient than reading multiple characters at once using other methods such as StreamReader.Read(char[], int, int) or StreamReader.ReadLine().
StreamReader.readline() method
The StreamReader.ReadLine() method is a method of the StreamReader class in C#, and it reads a line of characters from the current stream and returns the data as a string. A line is defined as a sequence of characters terminated by a carriage return character ('\r'), a newline character ('\n'), or a carriage return character followed immediately by a newline character.
Syntax:Following is an example of how you can use the StreamReader.ReadLine() method to read all the lines from a file:
In the above example, first create a StreamReader object that reads from the file "file.txt". Then, use a while loop to read each line from the file using the ReadLine() method. Inside the loop, do something with the line (in this case, simply write it to the console), and then read the next line.
Note that the StreamReader.ReadLine() method reads a whole line at a time, so it can be more efficient than reading single characters using the StreamReader.Read() method. However, it also means that if a line is very long, it may use a lot of memory to store the whole line as a string.
StreamReader.readtoend() method
StreamReader.ReadToEnd() method is a method of the StreamReader class in C# that reads the entire contents of a stream into a string.
The method returns a string that contains all the characters read from the stream.
Syntax:where reader is a StreamReader object that represents the stream you want to read.
The ReadToEnd() method reads from the current position in the stream until the end of the stream is reached. The method will block until the entire stream is read, so it's important to use it with care, especially with large streams.
Following is an example of how to reads the entire contents of a stream into a string.
In the above example, create a StreamReader object by passing the name of the file you want to read as a parameter to the constructor. Then use the ReadToEnd() method to read the entire contents of the file into a string variable. Finally, display the contents of the file on the console.
StreamReader.peak() method
The StreamReader.Peek() method is a method of the StreamReader class in C#, and it returns the next available character without actually reading it from the stream. The method returns an integer value that represents the Unicode code point of the next available character, or -1 if no more characters are available.
Syntax:Following is an example of how you can use the StreamReader.Peek() method to check the next available character in a file:
In the above example, first create a StreamReader object that reads from the file "file.txt". Then, use the Peek() method to check the next available character in the file without actually reading it from the stream. If there is a next character available, write a message to the console that shows the character. Otherwise, write a message that says there are no more characters available.
StreamReader.readblock() method
The StreamReader.ReadBlock() method is a method available in the .NET Framework that reads a block of characters from the current stream and writes the data to a buffer.
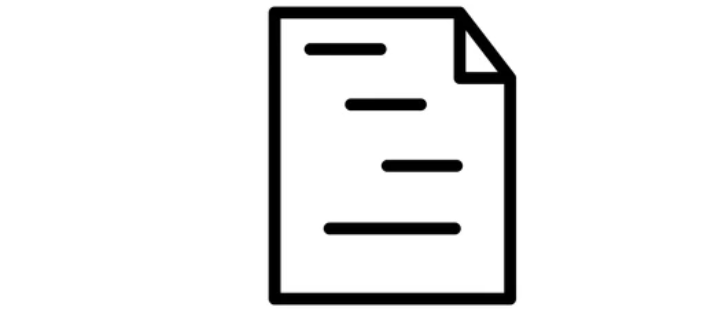
The method takes two parameters:
- buffer: An array of characters to write the data to.
- count: The maximum number of characters to read.
The method reads characters from the current stream until it has read the specified number of characters or until it reaches the end of the stream. The method then writes the characters it has read to the specified buffer.
Following is an example of how to use the StreamReader.ReadBlock() method to read a block of characters from a file:
In the above example, create a StreamReader object to read from a file called "myfile.txt". Then create a buffer to hold the characters that you read from the file. Call the ReadBlock() method to read a block of characters from the file into the buffer, and then print out the characters that were read. Finally, close the StreamReader.
Read webpage content using C# Streamreader
To read the content of a webpage in C# using StreamReader, you can follow these steps:
- Create a new WebRequest object using the WebRequest.Create() method and pass in the URL of the webpage you want to read as a string.
- Call the GetResponse() method on the WebRequest object to retrieve a WebResponse object that contains the response from the webpage.
- Get the Stream object from the WebResponse object using the GetResponseStream() method.
- Create a new StreamReader object and pass in the Stream object as a parameter.
- Use the StreamReader.ReadToEnd() method to read the entire contents of the webpage as a string.
Following is an example of how to read the content of a webpage using StreamReader in C#:
In the above example, first define the URL of the webpage you want to read as a string. Then create a new WebRequest object using the WebRequest.Create() method and pass in the URL. Retrieve a WebResponse object using the GetResponse() method on the WebRequest object, and then get the Stream object from the WebResponse object using the GetResponseStream() method. Create a new StreamReader object and pass in the Stream object, and then read the entire contents of the webpage using the StreamReader.ReadToEnd() method. Finally, close the StreamReader and WebResponse objects and print out the content of the webpage.
Reading from a Socket using C# StreamReader
Following is an example program that uses StreamReader to read data from a Socket in C#:
In the above example, first create a new Socket object and connect it to a server at 127.0.0.1:8080. Then get the NetworkStream from the socket and create a new StreamReader object, passing in the NetworkStream.
Then call the ReadToEnd() method on the StreamReader object to read all the data from the socket. Finally, display the data on the console and close the StreamReader, NetworkStream, and Socket objects.
- Advantages of C#
- C# vs. Java: What Are Its Main Differences
- Advantages of C# over Python
- First C# Program | Hello World
- Difference between Console.Write and Console.WriteLine in C#
- How do I create a MessageBox in C#?
- C# Comments
- How to reverse a string in C#
- Palindrome in C# with Examples
- Fibonacci Series Program in C# with Examples
- C# Program to Print Number Triangle
- Get Integer Input from User in C# Console Application
- C# StringBuilder | Learn To Use C# StringBuilder Class
- C# HashMap (Dictionary)
- Ternary Operator (? :) in C# with Examples
- How To Sort Datatable in C#
- Struct Vs Class in C# | Differencees and Advantages
- Async And Await In C#
- IEnumerable in C# | Examples
- ref Vs out in C#
- How to remove item from list in C#?
- How to Add Items to a C# List
- C# StreamWriter Examples
- C# Map Example
- Static Method In C# | Examples
- How to convert an Enum to a String in C#
- How to convert a string to an enum in C#
- How to filter a list in C#?