Console.WriteLine() and Console.Write() in C#?
A C# console program is a program that runs in a console or command-line interface, where the user interacts with the program by typing commands and receiving text output. C# Console programs are useful for a wide range of applications, such as system utilities, file management, and data processing.
C# console program
Following is an example of a simple C# console program that demonstrates the use of Console.Write() and Console.WriteLine():
This program prompts the user to enter their name, reads the input from the console using Console.ReadLine(), and then outputs a greeting message that includes the entered name using Console.WriteLine().
Let's take a closer look at the Console.Write() and Console.WriteLine() methods:
Console.Write()
This method writes a specified string to the console without a newline character at the end. In the example above, Console.Write("Enter your name: "); displays the prompt "Enter your name: " to the console without adding a newline character.
Console.WriteLine()
This method writes a specified string to the console followed by a newline character. In the example above, Console.WriteLine("Hello, " + name + "!"); writes the greeting message "Hello, [name]!" to the console, where [name] is the value entered by the user, and adds a newline character at the end.
Difference | Console.Write() and Console.WriteLine()
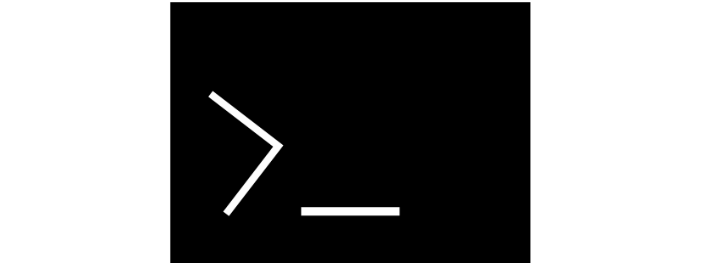
The difference between Console.Write() and Console.WriteLine() is that Console.Write() keeps the cursor on the same line after the text is written, while Console.WriteLine() moves the cursor to the next line. In our example, this means that the prompt and the user input are displayed on the same line, while the greeting message is displayed on a new line.
Overall, Console.Write() and Console.WriteLine() are useful methods for writing text to the console in C# console programs. They provide a flexible and customizable way to interact with users and display output.
- Advantages of C#
- C# vs. Java: What Are Its Main Differences
- Advantages of C# over Python
- First C# Program | Hello World
- How do I create a MessageBox in C#?
- C# Comments
- How to reverse a string in C#
- Palindrome in C# with Examples
- Fibonacci Series Program in C# with Examples
- C# Program to Print Number Triangle
- Get Integer Input from User in C# Console Application
- C# StringBuilder | Learn To Use C# StringBuilder Class
- C# HashMap (Dictionary)
- Ternary Operator (? :) in C# with Examples
- How To Sort Datatable in C#
- Struct Vs Class in C# | Differencees and Advantages
- Async And Await In C#
- IEnumerable in C# | Examples
- ref Vs out in C#
- How to remove item from list in C#?
- How to Add Items to a C# List
- C# StreamWriter Examples
- StreamReader in C# |Examples
- C# Map Example
- Static Method In C# | Examples
- How to convert an Enum to a String in C#
- How to convert a string to an enum in C#
- How to filter a list in C#?