What is a static method in C#?
In C#, a static method is a method that belongs to a class, rather than to any specific instance of the class. This means that you can call a static method on the class itself, without needing to create an object of that class first. Static methods are useful for defining functionality that is not dependent on any specific object's state.
C# static method
To define a static method in C#, you use the static keyword in the method signature.
In the above example, the Add method is declared as static by using the static keyword. This means that you can call Calculator.Add(x, y) to add two integers together, without needing to create an instance of the Calculator class.
Following is another example, this time using a static method to keep track of the number of objects that have been created from a particular class:
In the above example, the MyClass class has a static variable count that keeps track of how many instances of the class have been created. The constructor of the class increments this count each time a new object is created. The GetCount method is declared as static, so you can call MyClass.GetCount() to get the current count of objects created, without needing to create an instance of the class.
Static and non-static
One important thing to note about static methods is that they cannot access instance members of the class (i.e. non-static fields or methods). This is because static methods are not tied to any specific instance of the class, and therefore cannot access the state of any particular object. If you need to access instance members from a static method, you will need to pass in an instance of the class as a parameter.
Rules for static method in C#
Following are some important rules to keep in mind when working with static methods in C#:
Static methods can only access other static members:
Since static methods are not associated with any specific instance of a class, they can only access other static members of the class, including other static methods, fields, and properties.
Static methods cannot access instance members:
Static methods cannot directly access non-static (instance) members of a class, such as instance fields or instance methods. If you need to access instance members from a static method, you must pass an instance of the class as a parameter.
Static methods cannot be overridden:
Static methods are implicitly sealed, which means that they cannot be overridden in derived classes. If you want to provide an extensible behavior for static methods, you can use interfaces or abstract classes.
Static methods are called on the type, not the instance:
Static methods are called on the class itself, not on any particular instance of the class. You can call a static method on the type by using the class name, followed by the method name, for example: MyClass.StaticMethod()
Static methods can be used to create utility classes:
Static methods are commonly used to create utility classes, which provide a collection of methods that are not associated with any specific instance of a class. For example, the Math class in C# provides a collection of static methods for performing common mathematical operations.
Static methods can be used to initialize static fields:
You can use a static constructor or a static method to initialize static fields of a class. The static constructor is called only once, when the class is first accessed, while a static method can be called multiple times to initialize the static fields.
When and How to use C# static methods
Following are some common scenarios where you may want to use C# static methods:
- Utility functions: If you have a function that doesn't require any instance variables or state, you can make it a static method. For example, a mathematical function that calculates the square root of a number could be a static method.
- Factory methods: When you need to create instances of a class, you can use static methods to create them. This is often used in factory design patterns, where the factory class has static methods that create different types of objects.
- Extension methods: C# allows you to define extension methods that extend the functionality of existing classes. These methods are defined as static methods and can be called as if they were instance methods of the class they are extending.
- Singleton pattern: A common design pattern is the singleton pattern, where you want to ensure that only one instance of a class is created. You can use a static method to create the instance and make it a singleton.
- Performance optimization: In some cases, using a static method can provide a performance benefit. Because static methods don't require an object to be created, they can be faster to call than instance methods.
Static Class, static Fields and static Constructors in C#
Static Class, Static Fields, and Static Constructors are different types of static members in C#.
Static Class
A static class is a class that can only contain static members. Static classes cannot be instantiated, and they are sealed by default, which means that they cannot be inherited by other classes. A static class is useful when you want to provide a collection of related methods that do not depend on any particular instance of a class.
In the above example, the MathUtils class is marked as static, which means that it can only contain static members. The class contains a static field PI and a static method Add, which can be called directly on the class without the need to create an instance of the class.
Static Fields
A static field is a field that belongs to the class, rather than to any specific instance of the class. Static fields are initialized only once, when the class is first loaded into memory, and their values are shared across all instances of the class. Following is an example of a class with a static field:
In the above example, the Counter class has a static field count, which is incremented each time a new instance of the class is created. The GetCount method is marked as static, so you can call it directly on the class without the need to create an instance of the class.
Static Constructors
A static constructor is a special type of constructor that is called only once, when the class is first loaded into memory. Static constructors are used to initialize static fields or perform other initialization tasks that need to be done only once. Following is an example of a class with a static constructor:
In the above example, the MyLogger class has a static constructor that initializes a static field writer, which is used to write log messages to a file. The static constructor is called only once, when the class is first loaded into memory, and it creates the StreamWriter object and sets its AutoFlush property to true. The Log method is marked as static, so you can call it directly on the class without the need to create an instance of the class.
Static keyword in programming language
The static keyword is used in programming languages to specify that a member or method belongs to the class itself, rather than to any specific instance of the class.
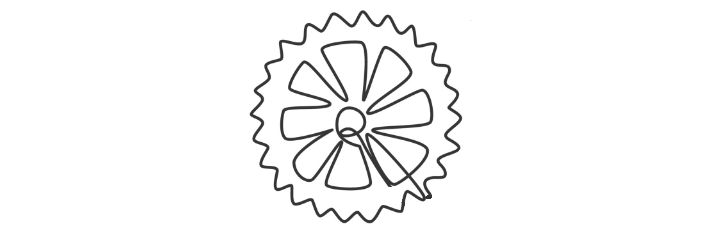
In object-oriented programming, a class is a blueprint for creating objects, and each object created from a class has its own set of instance variables and methods. However, there are cases where you may want to define members or methods that belong to the class itself, rather than to any particular instance of the class. This is where the static keyword comes in.
When a member or method is marked as static, it means that it belongs to the class, rather than to any particular object created from the class. This means that you can access the member or method directly on the class itself, without the need to create an instance of the class.
Some common uses of the static keyword include:
- Defining constants or configuration values that are shared across all instances of a class.
- Creating utility classes that provide a collection of related methods that do not depend on any particular instance of a class.
- Defining methods that perform operations that do not require access to instance variables or methods of a class.
Note that the use of static members and methods can affect the way that objects are created and used in your program, so it's important to use them wisely and only when they are appropriate for your particular use case.
- Advantages of C#
- C# vs. Java: What Are Its Main Differences
- Advantages of C# over Python
- First C# Program | Hello World
- Difference between Console.Write and Console.WriteLine in C#
- How do I create a MessageBox in C#?
- C# Comments
- How to reverse a string in C#
- Palindrome in C# with Examples
- Fibonacci Series Program in C# with Examples
- C# Program to Print Number Triangle
- Get Integer Input from User in C# Console Application
- C# StringBuilder | Learn To Use C# StringBuilder Class
- C# HashMap (Dictionary)
- Ternary Operator (? :) in C# with Examples
- How To Sort Datatable in C#
- Struct Vs Class in C# | Differencees and Advantages
- Async And Await In C#
- IEnumerable in C# | Examples
- ref Vs out in C#
- How to remove item from list in C#?
- How to Add Items to a C# List
- C# StreamWriter Examples
- StreamReader in C# |Examples
- C# Map Example
- How to convert an Enum to a String in C#
- How to convert a string to an enum in C#
- How to filter a list in C#?