How to Remove C# List Items
Following are some ways to remove items from a C# List:
- Remove method
- RemoveAt method
- RemoveAll method
- Clear method
- RemoveRange method
Using the Remove method:
The Remove method is used to remove a specific item from the list. It takes the object to be removed as a parameter, and removes the first occurrence of the item from the list. If the item is not found, nothing happens.
In the above example, the item "green" is removed from the list.
Using the RemoveAt method:
The RemoveAt method is used to remove an item at a specific index in the list. It takes the index of the item to be removed as a parameter.
In the above example, the item at index 1 (which is "blue") is removed from the list.
Using the RemoveAll method:
The RemoveAll method is used to remove all items from the list that match a certain condition. It takes a predicate as a parameter, which is a function that returns true or false for each item in the list.
In the above example, all even numbers are removed from the list.
Using the Clear method:
The Clear method is used to remove all items from the list. It takes no parameters.
In the above example, all items are removed from the list.
Using the RemoveRange method:
The RemoveRange method is used to remove a range of items from the list. It takes the starting index of the range and the number of items to remove as parameters.
In the above example, the range of items from index 1 to 3 (which are "green", "blue", and "yellow") is removed from the list.
Possible Exceptions | Remove C# List Items
When removing items from a C# list, there are a few possible exceptions that can be thrown:
- ArgumentOutOfRangeException: This exception is thrown if the index provided to remove the item is out of the valid range of indices for the list.
- ArgumentNullException: This exception is thrown if the item passed to remove is null, and the list does not allow null values.
- NotSupportedException: This exception is thrown if the list is read-only or fixed size and you try to remove an item from it.
To avoid these exceptions, you can do the following:
- Make sure the index you are trying to remove is within the valid range of indices for the list.
- Check if the item you want to remove is null and either remove it if the list allows null values or handle it appropriately.
- If you are working with a read-only or fixed-size list, consider creating a new list and copying the elements you want to keep to the new list, or using a different data structure that supports removing items.
Following is an example of removing an item from a list and handling possible exceptions:
In the above example, attempt to remove the item at index 2 from the list. If an exception is thrown, catch it and print an error message indicating which type of exception occurred and the message associated with it.
List in C#
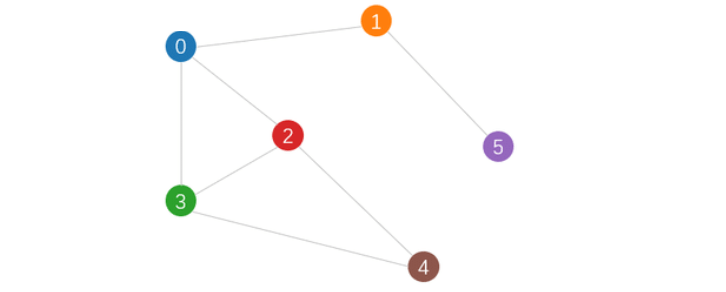
In C#, a List is a collection class that provides a dynamic, resizable array for storing and manipulating a set of elements. It allows adding, removing, and accessing items based on their index position. The List class is part of the System.Collections.Generic namespace and provides many useful methods for working with lists, such as sorting, searching, and filtering. Lists are commonly used in C# programming to store and manage sets of related data, such as user profiles, products, or orders.
- Advantages of C#
- C# vs. Java: What Are Its Main Differences
- Advantages of C# over Python
- First C# Program | Hello World
- Difference between Console.Write and Console.WriteLine in C#
- How do I create a MessageBox in C#?
- C# Comments
- How to reverse a string in C#
- Palindrome in C# with Examples
- Fibonacci Series Program in C# with Examples
- C# Program to Print Number Triangle
- Get Integer Input from User in C# Console Application
- C# StringBuilder | Learn To Use C# StringBuilder Class
- C# HashMap (Dictionary)
- Ternary Operator (? :) in C# with Examples
- How To Sort Datatable in C#
- Struct Vs Class in C# | Differencees and Advantages
- Async And Await In C#
- IEnumerable in C# | Examples
- ref Vs out in C#
- How to Add Items to a C# List
- C# StreamWriter Examples
- StreamReader in C# |Examples
- C# Map Example
- Static Method In C# | Examples
- How to convert an Enum to a String in C#
- How to convert a string to an enum in C#
- How to filter a list in C#?