C# Ternary Operator (? :)
The ternary operator in C# is a shorthand notation for an if-else statement that takes three operands, hence its name "ternary". It is commonly used to evaluate a condition and assign a value based on whether the condition is true or false. The syntax of the ternary operator is as follows:
The condition is evaluated first, and if it is true, the expression before the colon (:) is executed. If the condition is false, the expression after the colon is executed. The result of the ternary operator is the value of the expression that is executed.
Following are some examples of using the ternary operator in C#:
Assigning a value based on a condition
Using a ternary operator in a method call
Nested ternary operators
In the above example, the first ternary operator checks if x is greater than 0. If it is, a second ternary operator is used to check if x is less than 10. If it is, the result is "small positive number", otherwise it is "positive number". If x is not greater than 0, the result is "negative number".
Advantages of ternary operator in c#
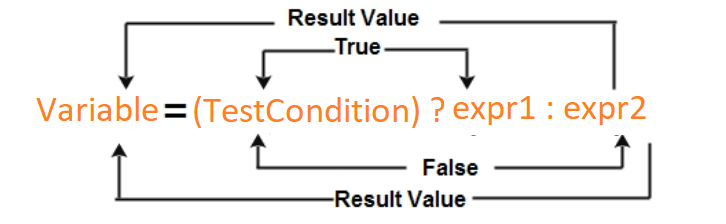
The ternary operator in C# offers several advantages over traditional if-else statements. Here are some of the key advantages:
- Concise syntax: The ternary operator allows you to write conditional expressions with a concise and easy-to-read syntax. This can help to reduce code clutter and make your code more readable.
- Fewer lines of code: Using the ternary operator can help you to write shorter and more compact code, which can be easier to maintain and modify in the future.
- Improved performance: In some cases, the ternary operator can offer better performance than if-else statements. This is because it can be more efficient to evaluate a single expression than to execute a series of branching statements.
- Expressive code: Using the ternary operator can help to make your code more expressive and self-documenting. By using a concise and clear syntax, you can make it easier for others to understand your code and its intent.
- Easier to debug: When you use the ternary operator, it can be easier to debug your code, as you can easily see the condition and the expressions that are being evaluated. This can help you to identify errors and fix them more quickly.
When to use ternary operator in C#
The ternary operator in C# is a useful tool for writing concise and readable code. However, it may not always be the best choice for every situation. Here are some factors to consider when deciding whether to use the ternary operator:
Simple conditions:
The ternary operator is best suited for simple conditions that can be expressed in a single line of code. If the condition involves complex logic or multiple variables, it may be better to use an if-else statement.
Single assignments:
The ternary operator is ideal for assigning a single value based on a condition. If you need to perform multiple assignments or execute multiple statements based on a condition, it may be better to use an if-else statement.
Readability:
The ternary operator can help to improve the readability of your code, especially when used sensibly. If you find that an if-else statement makes your code harder to read or understand, the ternary operator may be a good choice.
Personal preference:
Ultimately, the decision to use the ternary operator is a matter of personal preference and coding style. If you prefer the concise syntax of the ternary operator and find it easy to read and understand, then it may be a good choice for you.
Conclusion:
There are no specific methods that are unique to the ternary operator, as it is simply a shorthand notation for an if-else statement. However, it can be used in a variety of ways to simplify code and make it more readable. By using this operator in the right situations, you can simplify your code, make it more efficient, and make it easier to read and maintain over time. However, it should be used wisely and with care, especially when dealing with complex conditions or multiple assignments.
- Advantages of C#
- C# vs. Java: What Are Its Main Differences
- Advantages of C# over Python
- First C# Program | Hello World
- Difference between Console.Write and Console.WriteLine in C#
- How do I create a MessageBox in C#?
- C# Comments
- How to reverse a string in C#
- Palindrome in C# with Examples
- Fibonacci Series Program in C# with Examples
- C# Program to Print Number Triangle
- Get Integer Input from User in C# Console Application
- C# StringBuilder | Learn To Use C# StringBuilder Class
- C# HashMap (Dictionary)
- How To Sort Datatable in C#
- Struct Vs Class in C# | Differencees and Advantages
- Async And Await In C#
- IEnumerable in C# | Examples
- ref Vs out in C#
- How to remove item from list in C#?
- How to Add Items to a C# List
- C# StreamWriter Examples
- StreamReader in C# |Examples
- C# Map Example
- Static Method In C# | Examples
- How to convert an Enum to a String in C#
- How to convert a string to an enum in C#
- How to filter a list in C#?