C# Hello World | Your First C# Program
Following is an explanation of the steps to create your first C# program "Hello World":
Install and set up the development environment
The first step is to install a C# development environment such as Visual Studio or Visual Studio Code. Once installed, open the development environment and create a new console application project.
Write the code
Once you have created the project, you can write the "Hello World" code. In C#, this is a very simple program that outputs the text "Hello, World!" to the console.
This code does the following:
- The using System; statement is used to include the System namespace, which contains the Console class that we will use to output the text.
- The class Program line defines a new class called Program.
- The static void Main() method is the entry point for the program, and it is where the program starts executing. It is a static method, which means that it can be called without an instance of the Program class.
- The Console.WriteLine("Hello, World!"); line outputs the text "Hello, World!" to the console.
Build and run the program
Once you have written the code, you can build and run the program. In Visual Studio, you can do this by clicking the "Start" button or by pressing F5. The program will run, and you will see the text "Hello, World!" printed to the console.
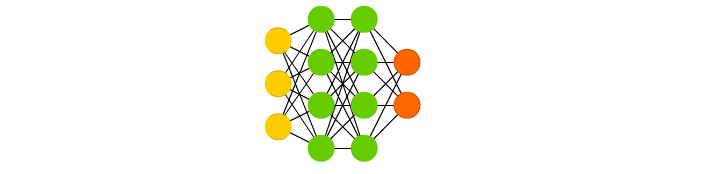
That's it! This is the basic structure of a C# console application, and it is a good starting point for learning the language.
- Advantages of C#
- C# vs. Java: What Are Its Main Differences
- Advantages of C# over Python
- Difference between Console.Write and Console.WriteLine in C#
- How do I create a MessageBox in C#?
- C# Comments
- How to reverse a string in C#
- Palindrome in C# with Examples
- Fibonacci Series Program in C# with Examples
- C# Program to Print Number Triangle
- Get Integer Input from User in C# Console Application
- C# StringBuilder | Learn To Use C# StringBuilder Class
- C# HashMap (Dictionary)
- Ternary Operator (? :) in C# with Examples
- How To Sort Datatable in C#
- Struct Vs Class in C# | Differencees and Advantages
- Async And Await In C#
- IEnumerable in C# | Examples
- ref Vs out in C#
- How to remove item from list in C#?
- How to Add Items to a C# List
- C# StreamWriter Examples
- StreamReader in C# |Examples
- C# Map Example
- Static Method In C# | Examples
- How to convert an Enum to a String in C#
- How to convert a string to an enum in C#
- How to filter a list in C#?